This tutorial is outdated. We're currently working on an updated version. In the meantime, for instructions on building a chat app using CometChat, please visit our documentation.
App and web development have come a long way over the last few years. We use a lot of chat applications every day, including Facebook Messenger, WhatsApp, Snapchat, and so on. One of the most widely used features is live voice chat. Using the voice chat sdk provided by cometchat and Firebase backend services you will learn how to make a voice chat app with Javascript with minimal effort.
*Note: For the full Javascript chat application, You can refer to this tutorial.
Follow along the steps to build a Javascript voice chat app that will provide:
1. A way for end-users to signup (email & password is sufficient)
2. A way for users to login in.
3. Use CometChat Chat Widget and configure it such that:
List of Users/Contacts is visible to all users with the search bar.
All users can initiate voice call each other.
4. Login the logged-in user to CometChat
5. Add API call when a user registers so that the user is created in CometChat
This tutorial will use Javascript, Firebase, and CometChat to build Javascript chat application.
Prerequisites
To follow this tutorial, you must have a degree of understanding of the general use of HTML, CSS, and Javascript to build the voice chat app. This will help you to improve your understanding of this tutorial.
Project Structure
To create a new project, you just need to create a folder which is called javascript-voice-chat-app. The image below reveals the project structure of our Javascript chat application. Make sure you see the folder arrangement before proceeding.
Project Structure.
Each subfolder and file will be explained in detail in the following section:
css: Contains all styling for our javascript chat application.
img: Contains images for the application such as logo, background, and so on.
js: Contains Javascript code and our business logic.
favicon.ico: It is favicon.ico for the application.
index.html: Root HTML file. HTML file for the home page.
login.html: HTML file for the login page.
.gitignore: This file contains files that will be ignored when committing the code. In this case, we do not want to commit the “config.js” file because it contains the secret keys of the Javascript chat application.
screenshots: This folder contains images that are used for the README.md file.
README.md: Describes the application and provides steps by steps to run the application.
Installing the Javascript Voice Chat App Dependencies
Step 1: You need to have Node.js installed on your machine.
Step 2: The application is using HTML, CSS, and Javascript. For this reason, you need to have a simple HTTP server to serve our application. In this case, you will use http-server, you can install the http-server by running the following statement. After installing the http-server package, you just need to “cd” to your project’s directory and run “http-server.”
npm install -g http-server
Configuring CometChat SDK
Head to CometChat Dashboard and create an account.
Register a new CometChat account if you do not have one
After registering a new account, you need to the log in to the CometChat dashboard.
Log in to the CometChat Dashboard with your created account
From the dashboard, add a new app called javascript-voice-chat-app
Create a new CometChat app - Step 1
Create a new CometChat app - Step 2
Select this newly added app from the list.
Select your created app
From the Quick Start copy the APP_ID, REGION, and AUTH_KEY, which will be used later.
Copy the the APP_ID, REGION, and AUTH_KEY
Navigate to the Users tab, and delete all the default users (very important).
Navigate to Users tab and delete all the default users
Navigate to the Groups tab and delete all the default groups (very important).
Navigate to Group tab and delete all the default groups
Create a file called config.js in the js folder of your project
Import and inject your secret keys in the config.js file containing your CometChat and Firebase in this manner.
const config = { apiKey: xxx-xxx-xxx-xxx-xxx-xxx-xxx-xxx, authDomain: xxx-xxx-xxx-xxx-xxx-xxx-xxx-xxx, databaseURL: xxx-xxx-xxx-xxx-xxx-xxx-xxx-xxx, projectId: xxx-xxx-xxx-xxx-xxx-xxx-xxx-xxx, storageBucket: xxx-xxx-xxx-xxx-xxx-xxx-xxx-xxx, messagingSenderId: xxx-xxx-xxx-xxx-xxx-xxx-xxx-xxx, appId: xxx-xxx-xxx-xxx-xxx-xxx-xxx-xxx, measurementId: xxx-xxx-xxx-xxx-xxx-xxx-xxx-xxx, CometChatAppId: xxx-xxx-xxx-xxx-xxx-xxx-xxx-xxx, CometChatRegion: xxx-xxx-xxx-xxx-xxx-xxx-xxx-xxx, CometChatAuthKey: xxx-xxx-xxx-xxx-xxx-xxx-xxx-xxx, CometChatAPIKey: xxx-xxx-xxx-xxx-xxx-xxx-xxx-xxx, CometChatWidgetId: xxx-xxx-xxx-xxx-xxx-xxx-xxx-xxx };
Make sure to include config.js in your .gitignore file from being exposed online.
Setting Up Firebase Project
According to the requirements of the Javascript voice chat app, you need to let users create a new account and login to the application, Firebase will be used to achieve that. Head to Firebase to create a new project and activate the email and password authentication service. This is how you do it:
To begin using Firebase, you’ll need a Gmail account. Head over to Firebase and create a new project.
Firebase
Firebase provides support for authentication using different providers. For example, Social Auth, phone numbers, as well as the standard email and password method. Since you will be using the email and password authentication method in this tutorial, you need to enable this method for the project you created in Firebase, as it is by default disabled.
Under the authentication tab for your project, click the sign-in method and you should see a list of providers currently supported by Firebase.
Firebase Authentication
Next, click the edit icon on the email/password provider and enable it.
Enable Firebase Authentication with Email and Password
Now, you need to go enable Firebase Realtime Database. We will Firebase Realtime Database to store the information of the users in the application. Please refer to the following part for more information.
Choose “Realtime Database” option
Click on “Create Database"
Select location where you realtime database will be stored
Select “Start in test mode” for the learning purpose
Please follow the guidance from Firebase. After following all steps, you will see the database URL. If you just need to update the databaseURL property in your config.js file with that value.
Database Url
On the other hand, your Firebase real-time database will be expired in the future. To update the rules you just need to select the “Rules” tab and update the read/write as you can see from the image below. Please do not forget to set the .indexOn for the “users” because we need to get the user’s information by email. Therefore, we need to make the query successful.
Database rules
On the project’s overview page, select the add app option and pick web as the platform. After finishing this step, you should see your Firebase credentials as follow, please update your .env file with your Firebase credentials.
Firebase credentials
Firebase Dashboard
Once you’re done registering the application, you’ll be presented with a screen containing your application credentials.
Data Structure - User
The above image describes the data structure of the users. A user will contain the following information:
avatar: the user’s avatar.
email: the user’s email.
id: the user’s id.
Configuring Styling for the Application
Inside our Javascript chat app project structure, you need to create a styles.css file inside the css folder and paste the codes here. The styles.css file will contain all CSS of the application.
Setting Up Images for the Application
To store images for the application such as the logo and other images, you need to create the img folder inside your javascript-voice-chat-app folder. Following that, if you can refer to this link to get the images that will be used in this tutorial.
Configuring the Firebase File
This firebase.js file is responsible for interfacing with Firebase authentication and database services. Also, it makes ready our email/password authentication service provider enabling us to sign in with email/password. Secret keys will be retrieved from the config file. As mentioned above, please do not share your secret keys on GitHub. Please create a file which is called firebase.js file inside the js folder.
The Login Page
The Login Page
In out Javascript voice chat app, this page is responsible for authenticating users using the Firebase authentication service. It accepts the user credentials and either signs him up or in, depending on if he is new to our application. To create the login page for the Javascript chat application, you need to follow the below steps:
*Note: The forgot password text is used to make the UI more attractive, we have not had the forgot password feature, yet.
Create login.html in the source folder. The source code can be found from here.
You need to include some third-party libraries such as CometChat Widget, Uuid, Validator, Firebase via CDN because you are building the Javascript chat app. Therefore, npm should not be used here. You need to add those libraries for some reasons:
CometChat Widget: help us to integrate CometChat Widget to the Javascript chat app. On the other hand, you need to use some other functionalities from CometChat such as registering a new user, letting the user log in to CometChat, or letting the user log out from CometChat.
UUID: each created user should have a unique id. Hence, the UUID library is used to generate a unique key for each account.
Validator: it is used to validate the login/sign-up form. Because you need to ensure that the input information is valid before creating a new account or letting a user log in to the chat application.
Firebase: it helps us to interact with Firebase and use Firebase services such as Firebase Authentication, Firebase Realtime Database, and so on.
Besides using third-party libraries, you need to build some files to handle the logic for the Javascript chat app such as auth.js, config.js, firebase.js, util.js, login.js, config.js and firebase.js were mentioned in the above sections. We will discuss auth.js, util.js, and login.js in the following parts.
The Auth.js File
According to the requirements of the JavaScript voice chat application, the application needs to provide a way for users to login in. After the user has logged in to the application, the user cannot go back to the login page. On the other hand, if the user has not signed in to the application, the user cannot go to the home page. We need to define a solution to handle this case. Therefore, auth.js will be created to help us achieve that. Please create the auth.js file inside the js folder.
The CometChat Widget provides the getLoggedinUser function to get the logged-in user. The auth.js file will be used on both the login page and the home page. It means that this file will be executed first before other actions. This file will take responsibility for checking whether the current user has logged in. If the user has not logged in to the application, the user would be redirected to the login page. Otherwise, if the current user has logged in to the JavaScript chat app, the user cannot go back to the login page.
The Util.js File
Some functionalities can be used across pages of the JavaScript voice chat app such as showing or hiding the loading indicator or get the information of the authenticated user. To avoid duplicated code, you should store all common functionalities in a single file, and in this case, this file is called util.js. Please create the util.js file inside the js folder. The full source code can found here.
The Login.js File
The file will take responsibility for handling the business logic for the login page. This file contains functions which can let the user register a new account or log in to the application. The full source code can be found from here.
After clicking the sign-up button, the registerNewAccount function will be triggered. It accepts a JSON object as a parameter and the JSON object contains the user’s information including the user’s email, user’s password, and user’s password confirmation. Before proceeding with further actions, the user’s information needs to be validated by using the validateNewAccount function. If the information is valid, the user’s avatar and user’s id will be generated. As mentioned before, each user should have a unique id. After that, the application will register a new account by using the Firebase Authentication service and then register an account on CometChat by using the CometChatWidget. You can refer to the below code snippet for more information.
To log in to the JavaScript chat application, the user needs to click on the Login button. The below code snippet describes how to handle the business logic for the sign-in feature. the user’s credentials will be taken from the input elements first and the application validates that information. If the input information is valid, the application will let the user sign in by using the Firebase Authentication Service and the CometChatWidget. Aside from that, the authenticated user will be redirected to the home page.
How to Create Chat Widget on CometChat
As mentioned above, you will learn how to make a voice chat app with JavaScript with minimal effort. It means you can achieve the full voice chat application with just a few clicks. You can achieve that because we have strong support from CometChat. Thanks to the CometChat team. They are providing great services. To create a chat widget on CometChat you need to follow the below steps:
Step 1: Go to the CometChat dashboard by using your created account and then go to your created app. Hopefully, you had one after finishing the Configuring CometChat section.
Go to your created CometChat application
Step 2: You need to choose the Chat Widget option on the left sidebar. You will be redirected to the page in which you can create a new chat widget.
Click on the Chat Widgets option
Step 3: On the current page, you need to choose the New Chat Widget option, CometChat system will create a new chat widget for you and redirect you to the created chat widget.
Click on the New Chat Widgets option
Step 4: You need to update your config.js with the following keys.
Update your config.js file with the corresponding keys
The CometChat dashboard provides a great way to help us configure all features for the JavaScript chat application with minimum effort. You just need to select the section that you want to customize and toggle those features by clicking on the “toggle” button. Aside from that, you can customize by adding your JavaScript code and CSS. The following images help you understand clearly what we are talking about.
Customize sections
Step 5: We need to turn off some features, because we just want to build a voice chat app, not a full chat application. At this step, please select the main settings section to customize its feature as follows.
Please select this section
Step 6: We expand the Core Chat section on the right side, and configure the options as follows
Configure the Core Chat section like this
As we can see, you don’t need to achieve the text chat feature. Therefore, we turn all of the options that are related to the text chat feature.
Step 7: We configure the Voice and Video Calling/Conferencing section like this.
Configure the Voice and Video Calling/Conferencing like this
Step 8: We need to configure the sidebar settings, we need to hide some options on the sidebar because we do not need them for the Javascript voice chat application.
Please select this section
Step 9: We need to configure the Navigation section as follows.
Configure the Navigation like this
As we can see, we just need to show the list of users, we do not need to have the text chat feature. Therefore, we will hide the chats section, and the groups section.
In conclusion, we can see that, we can achieve a Javascript voice chat application so easy with supports from the CometChat services, we can turn on/off features with just a few clicks. We do not need to develop everything from scratch.
The Home Page
The Home Page
After the user has logged in to the application, the user will be redirected to the home page and on this page, you will integrate CometChat Widget to build the JavaScript voice chat application. We have set up, created a new chat widget, and update the secret keys in the config.js file. It is time to integrate the created chat widget into the home page. To create the home page, you need to follow the below steps:
Step 1: Create index.html file in your project folder. The full source code can be found here.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta http-equiv="X-UA-Compatible" content="IE=edge" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Video Chat App with Vanilla JS</title> <link rel="stylesheet" href="/css/styles.css" /> <script defer src="https://widget-js.cometchat.io/v3/cometchatwidget.js" ></script> </head> <body> <div class="header"> <div class="header__left"> <h1>CometChat</h1> <div class="header__right header__right--hide" id="header__right"> <img src="" alt="Build Chat Widget with CometChat" id="user__image" /> <span id="user__name"></span> </div> </div> <span class="header__logout" id="header__logout" ><span>Logout</span></span > </div> <div id="cometchat"></div> <div id="loading" class="loading"> <div class="lds-roller"> <div></div> <div></div> <div></div> <div></div> <div></div> <div></div> <div></div> <div></div> </div> </div> <script src="/js/auth.js"></script> <script src="/js/config.js"></script> <script src="/js/util.js"></script> <script src="/js/index.js"></script> </body> </html>
As you can see on the code snippet above, you need to include the CometChat Widget from the CDN because we want to build the Javascript chat app by using JavaScript and CometChat Widget. You also need to include some common files, which were mentioned in the above sections, such as auth.js, config.js, util.js. The index.js file will be discussed in the following part.
The Index.js File
This file will take responsibility for showing greeting to the authenticated user on the header, handling the logic when clicking on the Logout button, and launching the CometChat Widget.
The full source code can be found from here.
The most important of the above code snippet is how to launch the CometChat Widget. Therefore, we can set up full features for the JavaScript voice chat application. According to the CometChat Widget documentation, you need to initialize the CometChat Widget first, if initialization is completed successfully, the code of launching the CometChat Widget will be executed. Aside from that, all configurations will be taken from the config.js file. You can refer to the below code snippet for more information. You can refer to the CometChat documentation for more information.
After clicking the “Logout” button, we should call the logout function which is provided by the CometChat team. If the function is executed successfully, we need to redirect the user to the login page. You can refer to the below code snippet.
Wrapping Up
In conclusion, we have done an amazing job in developing a JavaScript voice chat application by leveraging JavaScript, Firebase, and CometChat. You’ve been introduced to the chemistry behind the Javascript voice chat application and how the CometChat Widget makes chat applications buildable.
I hope you enjoyed this tutorial and that you were able to successfully build the JavaScript chat app. It's time to get busy and build other related applications with the skills you have gotten from this tutorial. You can start building your chat app for free by signing up to the CometChat dashboard.
For the full Javascript chat application, You can refer to this tutorial.
About the Author
Hiep Le is a software engineer. He takes a huge interest in building software products and is a full-time software engineer. Most of his work is focused on one thing - to help people learn.
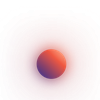
Hiep Le
CometChat