This tutorial is outdated. We're currently working on an updated version. In the meantime, for instructions on building a chat app using CometChat, please visit our documentation.
App and web development have come a long way over the last few years. We use a lot of rideshare sites every day, including Uber, Lyft, Grab, Gojek, and Be. One of the most widely used features is live chat. Using the CometChat communications SDK, Firebase backend services and Mapbox as OSRM service, you will learn how to build one of the best rideshare sites on the internet with minimal effort.
Follow along the steps to build an Uber clone that will allow users to request a ride. On the other hand, an user and a driver can talk to each other via text and voice calling. This tutorial will use React.js, Firebase, Mapbox and CometChat to build an Uber clone.
Prerequisites
To follow this tutorial, you must have a degree of understanding of the general use of React.js. This will help you to improve your understanding of this tutorial.
Installing the App Dependencies
Step 1: You need to have Node.js installed on your machine.
Step 2: Create a new project with the name uber-clone by running the following statement.
Step 3: You need to install some dependencies such as CometChat Pro, Firebase, Validator, Uuid, Leaflet, Leaflet-GeoSearch, Leaflet-Routing-Machine.
Configuring CometChat SDK
Head to CometChat and create an account.
Add real-time chat with minimal effort using CometChat
From the dashboard, add a new app called "uber-clone".
Select this newly added app from the list.
From the Quick Start copy the APP_ID, REGION, and AUTH_KEY, which will be used later.
Also, copy the REST_API_KEY from the API & Auth Keys tab.
Navigate to the Users tab, and delete all the default users and groups leaving it clean (very important).
Create a file called .env in the root folder of your project.
Import and inject your secret keys in the .env file containing your CometChat and Firebase in this manner.
9. Make sure to include .env in your gitIgnore file from being exposed online.
Setting Up Mapbox
In this project, we need to use the leaflet-routing-machine library to draw a route between two locations. However, the default OSRM server sometimes appears down. For this reason, we need another alternative solution. In this project, we will use Mapbox as an OSRM service. To setup Mapbox, you need to follow the below steps:
Head to Mapbox and create an account. After signing to Mapbox, you will be redirected to the page in which you will see the default public token.

Mapbox - Default Public Token
2. Update the .env file with Mapbox default public token.
Setting Up Firebase Project
Head to Firebase to create a new project and activate the email and password authentication service. This is how you do it:
To begin using Firebase, you’ll need a Gmail account. Head over to Firebase and create a new project.

Firebase
Firebase provides support for authentication using different providers. For example, Social Auth, phone numbers, as well as the standard email and password method. Since we’ll be using the email and password authentication method in this tutorial, we need to enable this method for the project we created in Firebase, as it is by default disabled.
Under the authentication tab for your project, click the sign-in method and you should see a list of providers currently supported by Firebase.

Firebase Authentication
Next, click the edit icon on the email/password provider and enable it.

Enable Firebase Authentication with Email and Password
Now, you need to go and register your application under your Firebase project. On the project’s overview page, select the add app option and pick web as the platform.

Firebase Dashboard
Once you’re done registering the application, you’ll be presented with a screen containing your application credentials. Take note of the second script tag as we’ll be using it shortly in our application.
Congratulations, now that you're done with the installations, let's do some configurations.
Configuring Styling for the Application
Inside your project structure, open the index.css files and paste the codes here. index.css file will contain all CSS of the application.
Initializing CometChat for the Application
The below codes initialize CometChat in your app before it spins up. The App.js file uses your CometChat API Credentials. We will get CometChat API Credentials from the .env file. Please do not share your secret keys on GitHub.
Actually, App.js does not contain only the above code. It also contains other business logic of the application. The full source code of App.js file can be found here.
Configuring the Firebase File
You need to create a “firebase.js” file inside the “src” folder and you need to enable Firebase realtime database. This file is responsible for interfacing with Firebase authentication and database services. Also, it makes ready our google authentication service provider enabling us to sign in with google. Secret keys will be stored in the .env file. As mentioned above, please do not share your secret keys on GitHub.
The below images demonstrate the data structure of the application. A user should have an avatar, an email, an id, a phone number, and a role (user role or driver role).
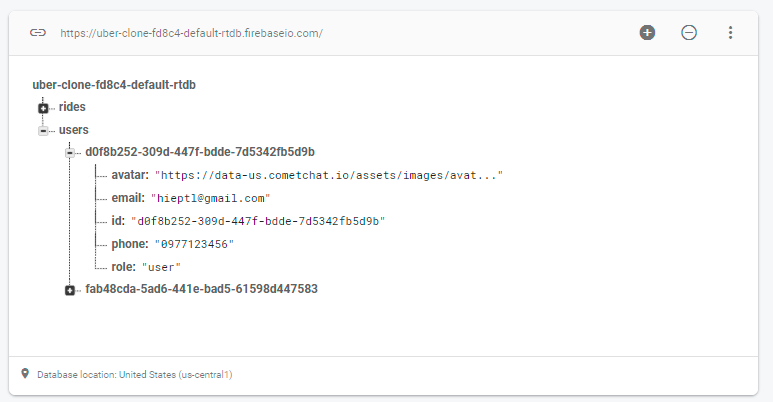
Data Structure - User
A ride should contain information of destination, pickup location, driver, requestor, and id of the ride. The status field demonstrates the status of the ride. If the status is 0, it means that a user is waiting for a driver. Following that, if the status is 1, it means that the ride has accepted by a driver. Aside that, if the status is -1, the ride has been canceled. The last but not least, if the status is 1, the ride has been finished.

Data Structure - Rides
Project Structure
The image below reveals the project structure. Make sure you see the folder arrangement before proceeding.
Now, let's make the rest of the project components as seen in the image above.

Project Structure
The App.js File
The App.js file is responsible for rendering different components by the given routes. For example, it will render the login page if the user has not logged in, yet or it renders the home page if the user has signed in to the system. On other hand, it will be used to initialize CometChat.
The full source code of the App.js file can be found here.
The Loading Component
The loading component will be shown when the system performs some side effects such as interacting with Firebase or calling CometChat APIs and so on. This component will be used to increase user experience. If we do not have this component, the end-users cannot know when the data is loaded successfully.
The full source code of the loading component can be found here.
The Login Component
This component is responsible for authenticating our users using the Firebase google authentication service. It accepts the user credentials and either signs him up or in, depending on if he is new to our application. See the code below and observe how our app interacts with Firebase and the CometChat SDK. The full source code can be found here.
The above code indicates that we are using withModal as a higher-order component. This higher-order component will be used to reuse the code of showing and hiding the custom modal. In this case, we want to show the sign-up modal to let end-users register new accounts. We will discuss the sign-up component in the following section.
The Sign Up Component
The sign-up component will help end-users to register new accounts. This component will do two things. The first thing is to register new accounts on Firebase by using the Firebase authentication service. Aside from that, it also registers new accounts on CometChat by using the CometChat SDK. The full source code can be found here.
The Home View
This is where all the magic happens. This component embodies other sub-components like the Header, Address Picker, Ride Detail, Ride List, etc.
As intuitive as they sound, the above sub-components can be best observed in the image below.

Home Component, Address Picker Component and Header Component

Request Ride Component

Ride List Component - The current user is a driver

Ride Detail Component - The current user is a driver
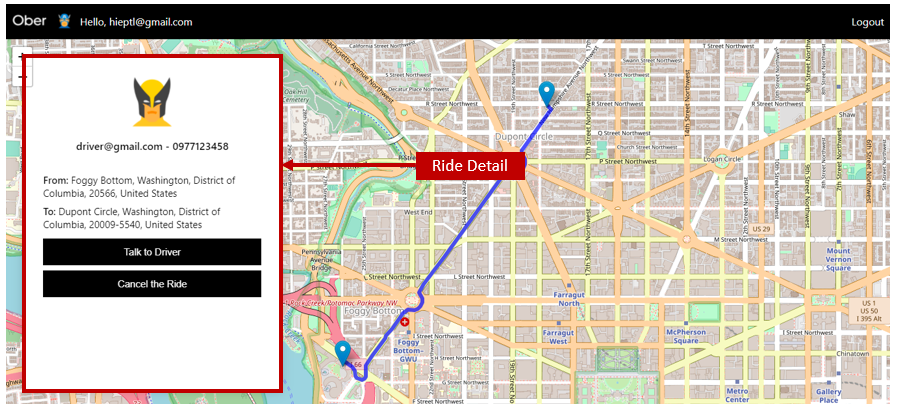
Ride Detail Component - The current user is a normal user

CometChat Messages Component

CometChat Messages Component - Voice and Video Calling
The Header Component
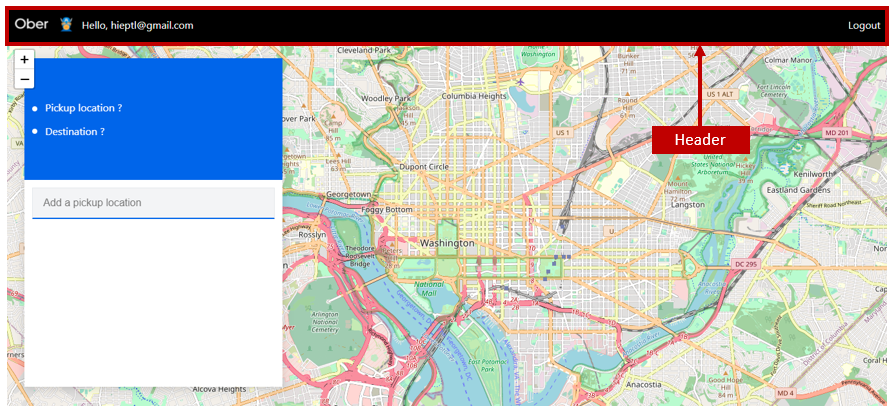
Header Component
The header component will be used to demonstrate the header of the page. It contains the logo the greeting and the logout button. The full source code of the header component can be found here.
The Address Picker Component

Address Picker Component
This component will allow the user to input the pickup location and the destination. The application will suggest locations to the user while the user is typing on the search box, The full source code can be found here.
The Request Ride Component

Request Ride Component
After inputting the pickup location and the destination, this component will be used to ask the user whether the user wants to request a ride, or not. If the user clicks on the “Change” button, the dialog will be closed. On the other hand, if the user clicks on the “Requesting a ride now” button, the application will find a driver for the user. The full source code can be found here.
The Ride List Component

Ride List Component - The current user is a driver
This component will be used to show a list of requesting rides, this component is just available for drivers. It means that if the current user is a driver, the user will see this component instead of the address picker component. The full source code can be found here.
The Ride Detail Component
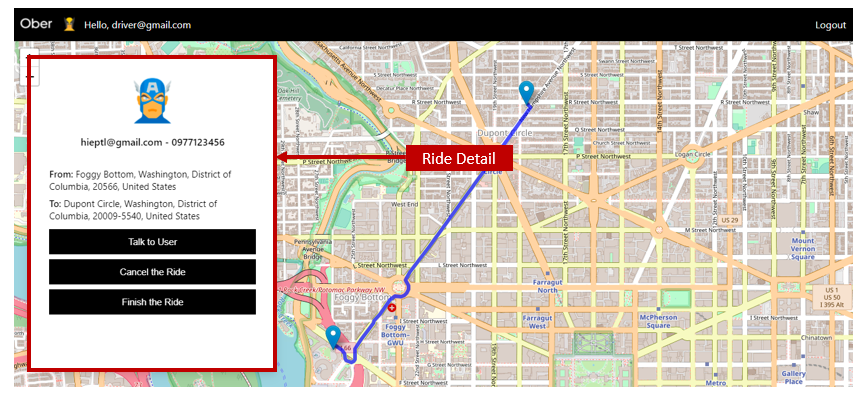
Ride Detail Component - The current user is a driver
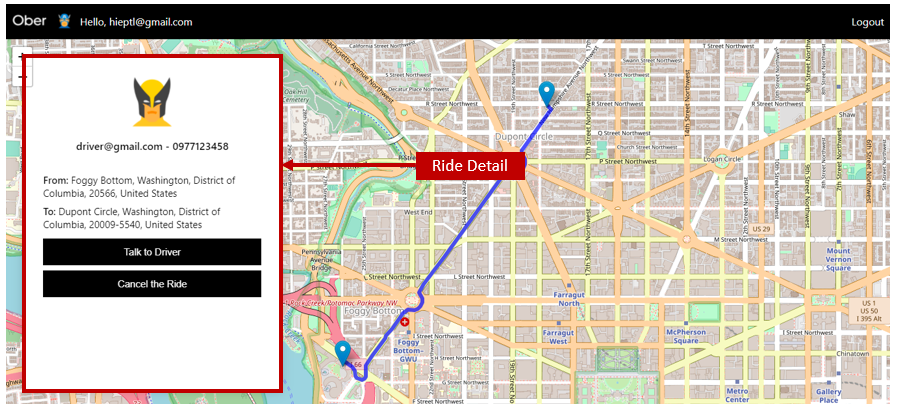
Ride Detail Component - The current user is a normal user
This component is used to help the driver and the user view information of each other. On the other hand, it provides some options such as canceling the ride, finishing the drive, talking to each other.
Add the CometChat UI to our Application
Before we can use the CometChat Pro React UI kit, we need to add it in our project so that we can reference it. In this case, we are using React UI Kit v3.0. To do that, follow the next steps:
Step 1: Clone the CometChat Pro React UI Kit Repository like so:
git clone https://github.com/cometchat-pro/cometchat-pro-react-ui-kit.git -b v3
Step 2: Copy the folder of the CometChat Pro React UI Kit you just cloned into the src folder of your project:

Copy of the cloned folder in to the src folder

React UI Kit Dependencies
Step 3: Copy all the dependencies from the package.json file of the CometChat Pro React UI Kit folder and paste them in the dependencies section of the package.json file of your project.
Step 4: Save the file and install the dependencies like so: npm install
As soon as the installation is completed, you now have access to all the React UI Components. The React UI kit contains different chat UI components for different purposes as you can see in the documentation here. It includes:
1. CometChatUI
2. CometChatUserListWithMessages
3. CometChatGroupListWithMessages
4. CometChatConversationListWithMessages
5. CometChatMessages
6. CometChatUserList
7. CometChatGroupList
8. CometChatConversationList
The Chat Component

CometChatMessages Component

CometChatMessages Component - Voice and Video Calling
In our Uber clone, we want to connect the user and the driver. It means that the user should be able to chat with the driver. Following that, the application should support text and voice calling. For this reason, we will create the Chat component and inside the Chat component, we use the CometChatMessages component from React UI Kit. The full source code can be found here.
Wrapping Up
In conclusion, we have done an amazing job in developing an Uber clone by leveraging React.js, Firebase, Mapbox, CometChat Pro SDK and CometChat React UI Kit. You’ve been introduced to the chemistry behind Uber and how the CometChat SDK makes rideshare applications buildable.
You have seen how to integrate most of the CometChat functionalities such as texting and real-time messaging. I hope you enjoyed this tutorial and that you were able to successfully clone Uber.
It's time to get busy and build other related applications with the skills you have gotten from this tutorial.
About the Author
Hiep Le is a software engineer. He takes a huge interest in building software products and is a full-time software engineer. Most of his work is focused on one thing - to help people learn.
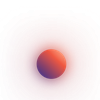
Hiep Le
CometChat