This tutorial is outdated. We're currently working on an updated version. In the meantime, for instructions on building a chat app using CometChat, please visit our documentation.
In this tutorial, I am going to guide you through building a clone of WhatsApp using the React Library. Don't worry! We will be leveraging CometChat to make things easy. So we will not be writing too much code. This is what we will be building, as shown in the image given below:

Demo of project to be built
Now, Let's hit the road...
Prerequisite
In order to be able to follow along, you need to have basic knowledge of React. If you will like to catch up on this, check out the React official Website: https://reactjs.org/.
Clarification of Terms
React
React is a JavaScript library for building user interfaces or UI components. It is open-source and is maintained by Facebook and a community of volunteers. It can be used as a base in the development of single-page websites. See more on the official Website: https://reactjs.org/.
CometChat
CometChat provides Text chat & video calling solutions for websites and applications to quickly connect your users with each other; patients with doctors, buyers with sellers, teachers with students, gamers, community users, event attendees, and more.
For this tutorial, we will be focusing on the CometChat Pro product. It houses highly customizable and easy-to-use SDKs, UI kits, Extensions and Plugins. It also supports more than 10 programming languages and platforms as you may see in the docs here.
With this information, you can see that what you can do with CometChat is only limited to your imagination. It could be solutions on Social community, Marketplace, Dating, On-Demand, Edu-Tech, Live Stream and so on. Just Dream and Build with the support of CometChat.
Messaging sites like WhatsApp _are now a common tool today as it has found its way to revolutionize communication. As a developer, you must have thought of the complexity of building an application that will provide such functionalities that WhatsApp provides. Functionalities such as adding our choice of contact and chatting with them is something that has made WhatsApp so unique.
_
Build the Starter App
We want to begin by building out the necessary parts of the application we need. We need a place to sign up, login, logout, add friend, and show chat UI. Follow the next steps to build that out:
Create a new react project with whatsapp-cometchat as the name npx create-react-app whatsapp-cometchat
In the src folder, create two files:
Register.js
Login.js.
Enter the following code in the Register.js file
Enter the following code in the Login.js file
Navigate to the App.js file and replace the existing code there with the following code:
In the code above, we are rendering the Chat Body and the Authentication Forms altogether. So if you view your project in the browser, you should see a whole lot going on. Shortly, we will make it make sense.
The following code will go into the App.css file to make the our app look a bit better
We now have a base for our project. Let's integrate CometChat
Integrate CometChat
In the section, we are going to be doing the following:
Create an account with CometChat and Create our first App on our CometChat dashboard.
Initialize CometChat in our project.
Dynamically render auth_forms and chat_body depending on the user's authentication status
Create a new user on our CometChat dashboard from our application
Login a user to our CometChat dashboard from our application
Add a friend to a user on our CometChat dashboard from our application
Logout a user from our CometChat dashboard from our application
Add the CometChat UI to our application chat body
Let's dive in...
STEP 1: Create An Account With CometChat and Create Our First App
Follow the next steps to quickly create a CometChat Pro account:
Signup and login to CometChat dashboard
Add real-time chat with minimal effort using CometChat

CometChat Pro Signup page
You should be on your dashboard like mine below:

CometChat Pro dashboard
Now, let's create an app to obtain a set of unique values we will be using in a moment
Create an App
In your dashboard, click on the Add New App button
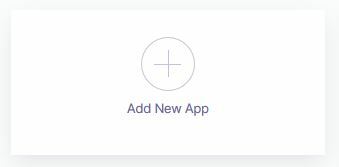
Add New App button
Fill out the screen that pops up and click on the Add App button

Create App Form
You should get this congratulatory popup. Click on the Get Started to be redirected to the dashboard of the app you just created

congratulatory popup
You should now be in that App's dashboard like so:

A CometChat App dashboard
All Good! Your App has been created.
STEP 2: Initialize CometChat In Our Project
Now let's invite CometChat into our project. The following steps will help us do that:
Install CometChat through the terminal like so: npm install @cometchat-pro/chat@latest --save
Navigate to the index.js file in your project and import CometChat like so: import { CometChat } from "@cometchat-pro/chat";
Since all other files are linked to index.js file, it is important you do the initialization in the index.js file so that other files have easy access to the initialization.
Enter the following code just after you have imported CometChat:
Do not forget to replace the APP_ID and the REGION with your own values. You can get yours from your dashboard. Click on the App you created and you should have the following details:

App Unique Keys
To see it working, save the file and check your browser's console
STEP 3: Dynamically Render auth_forms and chat_body Depending On The User's Authentication Status
At this point, we want to show the Login and Registration forms if the user is NOT logged in. If, on the other hand, the user is logged in, we will show them the chat body, the form to add friend and the logout button.
Navigate to the App.js file
Replace the code there with the following:
In the code above, we initialized a user constant and set it to null. We rendered logout button, add friend form, and chat if the user exists or is authenticated else we display the Login and Register forms. Then we called a logout function for our logout button and addFriend function for the Add Friend button. Finally, we passed down the setUser function as a props to the authentication forms so that the user will be updated with the setUser function as soon as login or registration is successful.
We will be back shortly to flesh out the empty functions on this page. Meanwhile, since we have not authenticated any user yet, if you view your application now, you should have the following screen:

Authentication Forms
STEP 4: Create New User On Our CometChat Dashboard From Our Application
It is now time to make it possible for users to join our application. Follow the next steps:
Navigate to the Register.js file
Replace the code there with the following:
In the code above, we imported CometChat at the top, initialized the input values and obtained the setUser function from the props we sent down from our App.js file, and we set up a handleSubmit function to be fired once the form is submitted.
Enter the following code in the handleSubmit function :
Do not forget to replace the AUTH_KEY with your own value.
In the code above, we are setting the uid to the phone number of the user because just like WhatsApp, two users cannot have the same phone number. Then we set the name to a combination of the userName and phone number because just like WhatsApp, we can save a user just how we like it to be.
Now just after the registration is successful, we want to log in the user. We will do that in the success block of the create user function above. The block I am referring to is this:
Do not forget to replace the AUTH_KEY with your own value.
Can you see how we have been able to use the uid to log in and used the setUser function to update the user indicating that the user is authenticated?
If you register a user now, you should be redirected to this screen:
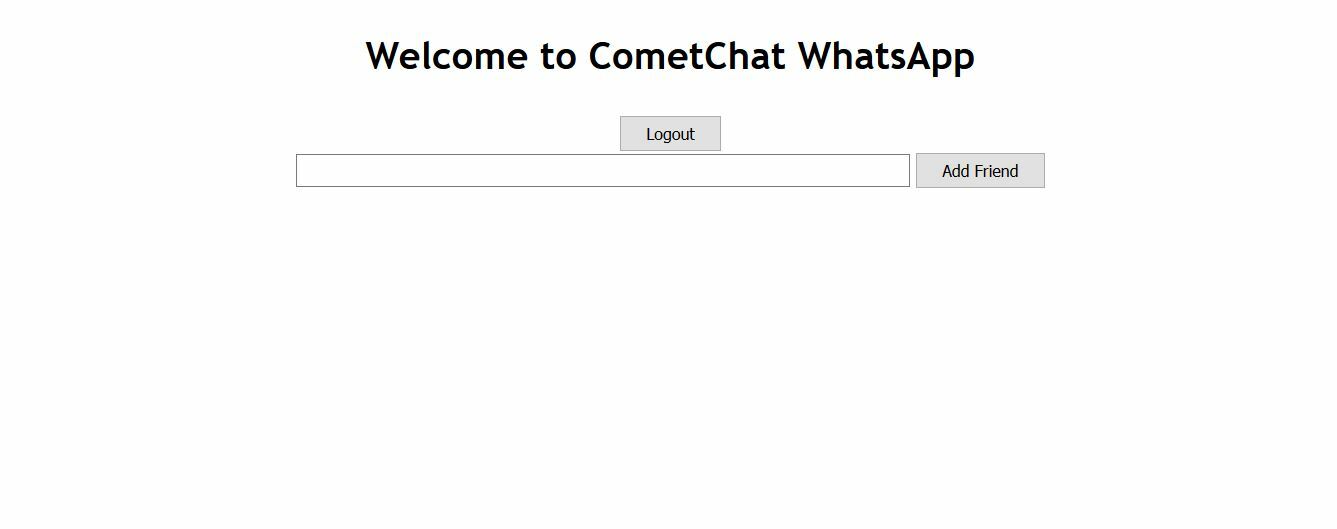
Logout and Add Friend Screen
Register in action
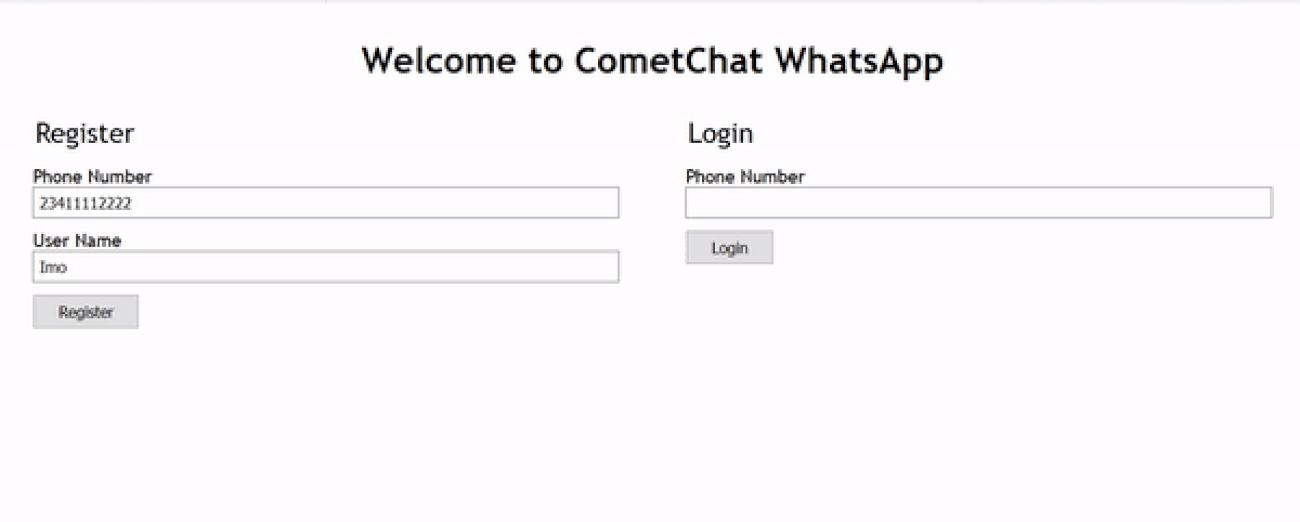
Register in action
STEP 5: Login A User To Our CometChat Dashboard From Our Application
If we have a returning user, then the returning user will have to use the login form since we can't register one user multiple times. So, to enable user login, do the following:
Replace the content of the Login.js with the following code:
In the code above, we have imported CometChat, received the setUser props and created an empty handleSubmit function that will be fired when the form is submitted.
In the handleSubmit function, enter the following code:
Do not forget to replace the AUTH_KEY with your own value.
With this, login is complete and you should be redirected to this screen once login is successful:
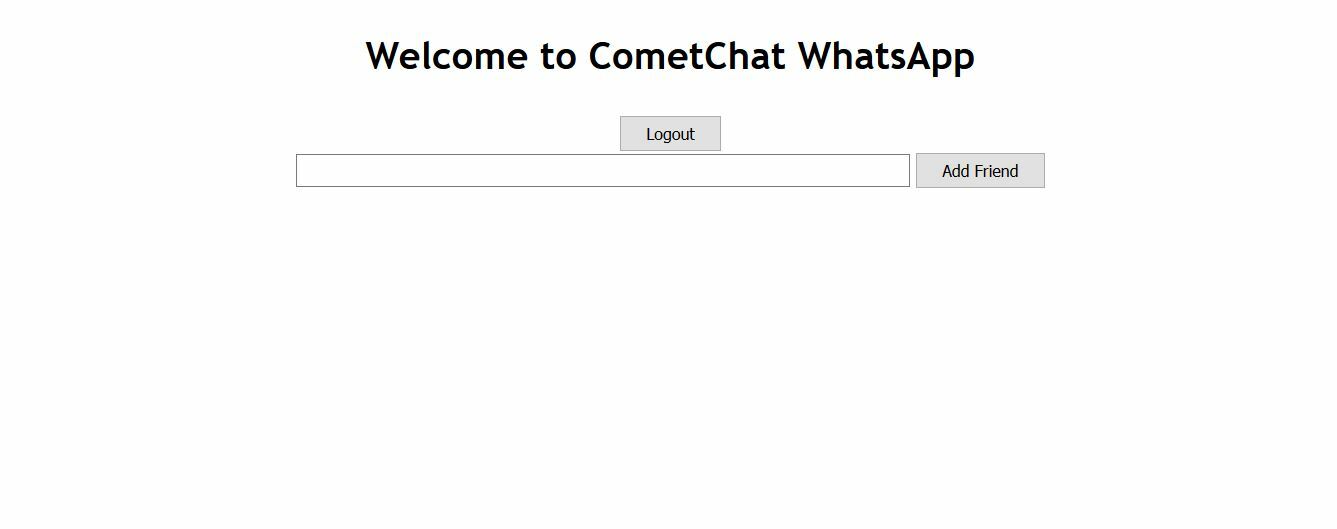
Logout and Add Friend Screen
STEP 6: Add A friend To A User On Our CometChat Dashboard From Our Application
Now that we can authenticate users, we need to make it possible for them to be able to chat with just their friends. Remember that on WhatsApp, for you to be able to chat with a friend, that person must be a registered user. It is the same with CometChat.
Before you add a new contact to your friends list, ensure that the contact is already registered on our application. So create a couple of contacts or users on your app so that you will have contacts to add as friends.
Now let's navigate to the App.js file to flesh out the addFriend function
Import CometChat at the top of the file like so: import { CometChat } from "@cometchat-pro/chat";
The following code will go into the addFriend function
Do not forget to replace the appId and apiKey with yours
In the code above, body: JSON.stringify({ accepted: [phone] }) collects an array of the contacts to be added as friends. Since we are going to be adding one friend at a time, we add just one phone number to the array.
You can now add any contact that has been created before as a friend
STEP 7: Logout A User From Our CometChat Dashboard From Our Application
Still in the App.js file, add the following code to the empty logout function:
Notice that we are setting the user to null as soon as logout is successful. This will hide chat body and display the authentication forms.
Login and Logout in action

Login and Logout in action
STEP 8: Add The CometChat UI To Our Application Chat Body
Before we can use the CometChat Pro React UI kit, we need to add it in our project so that we can reference it. To do that, follow the next steps:
Clone the CometChat Pro React UI Kit Repository like so: git clone https://github.com/cometchat-pro/cometchat-pro-react-ui-kit.git
Copy the folder of the CometChat Pro React UI Kit you just cloned into the src folder of your project:

React UI Kit Copied into the src folder
Copy all the dependencies from the package.json file of the CometChat Pro React UI Kit folder and paste them in the dependencies section of the package.json file of your project.
Save the file and install the dependencies like so: npm install
As soon as the installation is completed, you now have access to all the React UI Components. Walah!!!!
The React UI kit contains different chat UI components for different purposes as you can see in the documentation here. It includes:
1. CometChatUI
2. CometChatUserListWithMessages
3. CometChatGroupListWithMessages
4. CometChatConversationListWithMessages
5. CometChatMessages
6. CometChatUserList
7. CometChatGroupList
8. CometChatConversationList
But we are concerned with the component that will show us a list of users who are our friends and show us the conversations we might have had. The Chat UI Component is: CometChatUserListWithMessages.
The CometChatUserListWithMessages component displays a list of all the users in our application but we can limit it to display only our friends by adding the friendsOnly={true} props.
To make that happen, import it at the top of the App.js file like so:
You should have the following screen if you are a new user with no friends yet:

User Chat with no friends
If you have friends, your screen will look like this:
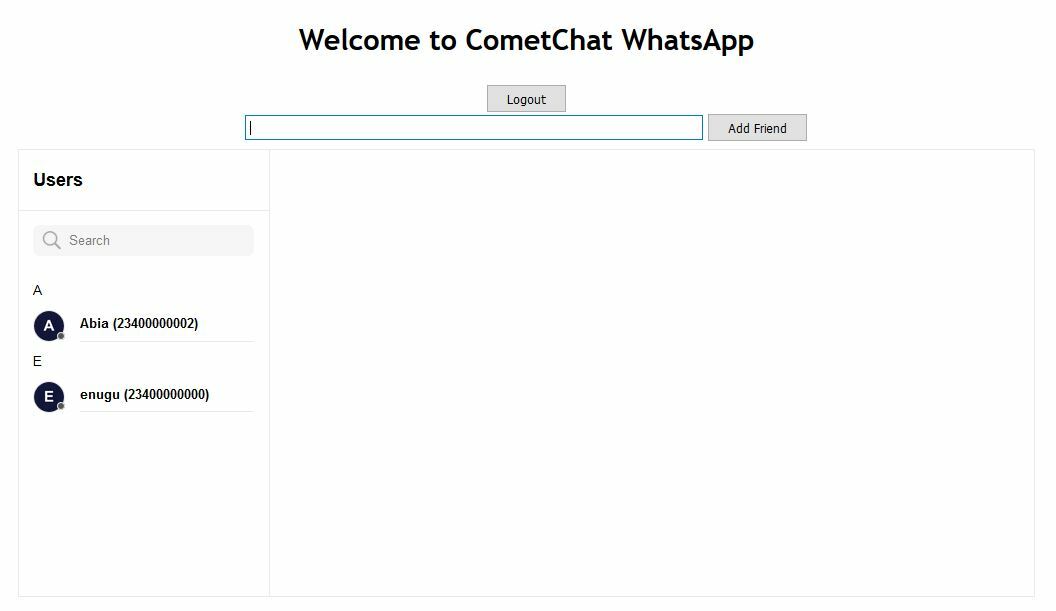
User Chat with friends
Add Friend(s) in action

Add Friend(s) in action
Friends Chat in action

Friends Chat in action
And that is it! We have created our own WhatsApp
Conclusion
We have been able to see the possibility of creating a WhatsApp-like application using React with the help of CometChat. We made functions to create new user, login user, add a friend, logout of the application and chat with friends in a very short while.
You will notice that the were eight (8) Chat UI Component but we only made use of one. Please do take time to check out the documentation and see what you can achieve with the other Components.
About Author
Njoku is a software engineer who is interested in building solutions to real world problems and teaching others about the things I know. Something I really enjoy doing asides writing codes and technical articles is body building.
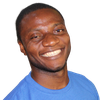
Njoku Samson Ebere
CometChat