This tutorial is outdated. We're currently working on an updated version. In the meantime, for instructions on building a chat app using CometChat, please visit our documentation.

A VueJs Tinder Clone
Introduction
As a software developer in this era, you can be sure that you'll face situations where you need to add a communication feature into an app. This tutorial will help you develop web-based communication solution for chatting and calling using CometChat. I will be guiding you step-by-step with no step missed, so get ready for a smooth ride.
Prerequisites
To follow this tutorial, you must have understood the fundamental principles of VueJs. This will speed up your comprehension of this tutorial.
Installing The App Dependencies
First, you need to have NodeJs installed on your machine, you can go to their website to do that.
Second, you need to also have the Vue-CLI installed on your computer using the command below.
npm install -g @vue/cli
Next, create a new project with the name tinder-clone and select the default preset.
vue create tinder-clone

VueJs Project Presets
Last, install these essential dependencies for our project using the command below.
_npm install vue-router vue-swing vue-material-design-icons firebase
_
Now that we're done with the installations, let's move on to building our tinder clone solution.
Installing CometChat SDK
Head to CometChat Pro and create an account.
From the dashboard, create a new app called "Chat Apps".
One created, click Explore.
Go to the API Keys tab and click Create API Key.
Create an API key called "Tinder Clone" with Full Access.
Click on the newly created API, navigate to the Users tab, and delete all the default users leaving it blank (very important).
Get the VueJs CLI installed on your machine by entering this command on your terminal. npm install -g @vue/cli
Create a ".env" file in the root directory of the project.
Enter your secret keys from CometChat and Firebase in this manner.
Duplicate the ".env" file and rename it to ".env".
Exclude ".env" and “.env.production” in the ".gitignore" file from getting exposed on Github.
Run the following command to install the CometChat SDK.
The Environment Variables
The setup below spells out the format for configuring the .env files for this project.
Setting Up Firebase Project
Head to Firebase create a new project and activate the email and password authentication service. This is how you do it.
To begin using Firebase, you’ll need a Gmail account. Head over to Firebase and create a new project.
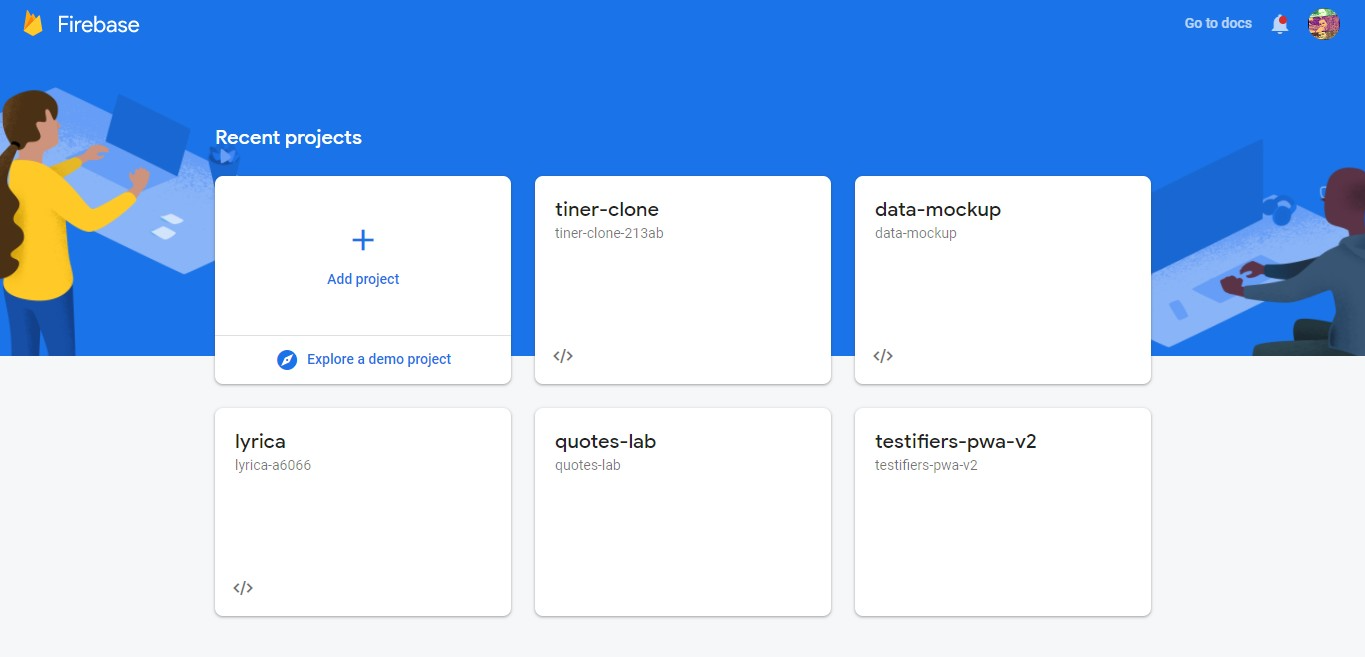
Firebase Console Create Project
Firebase provides support for authentication using different providers. For example Social Auth, phone numbers as well as the standard email and password method. Since we’ll be using the email and password authentication method in this tutorial, we need to enable this method for the project we created in Firebase, as it is by default disabled.
Under the authentication tab for your project, click the sign-in method and you should see a list of providers Firebase currently supports.

Firebase Authentication Options
Next, click the edit icon on the email/password provider and enable it.
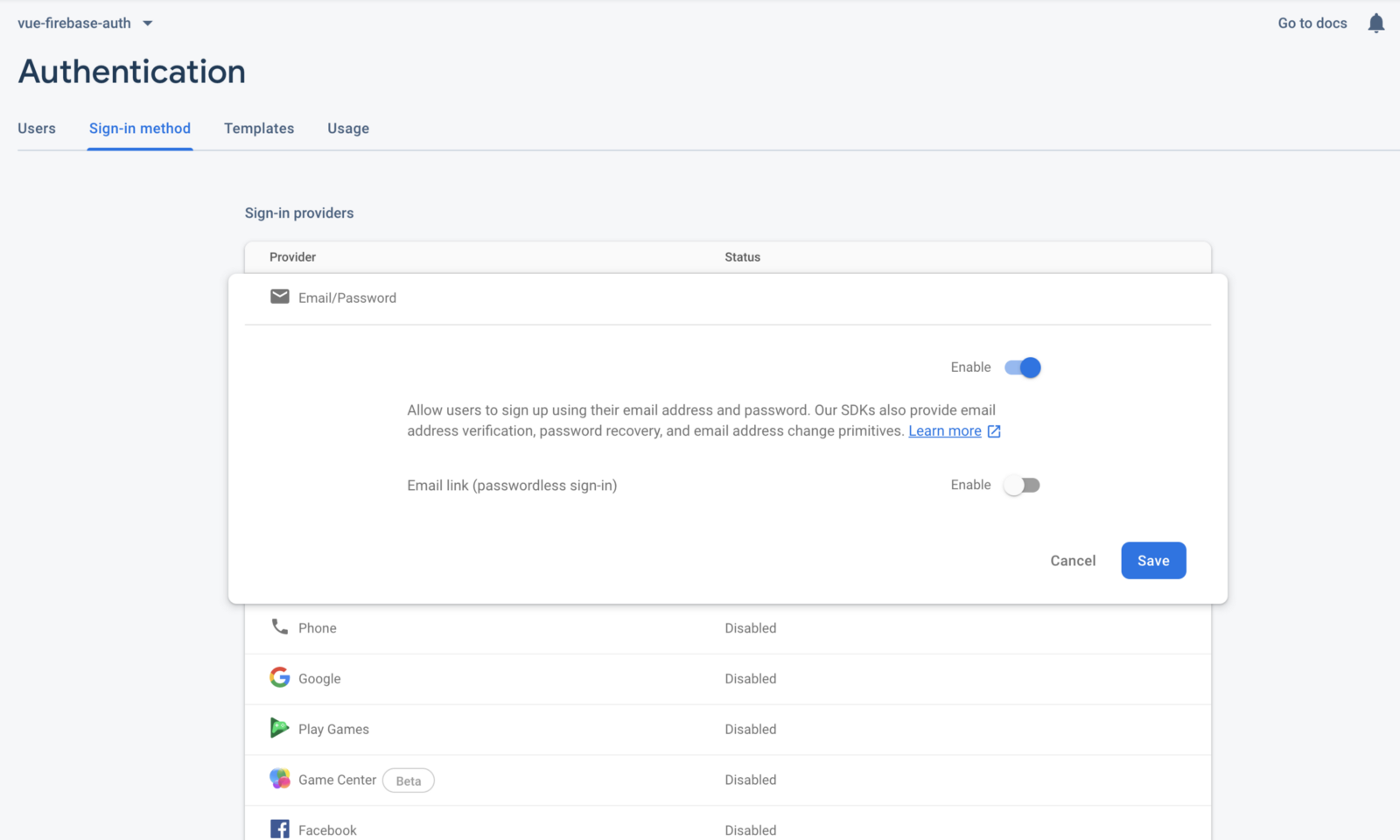
Firebase Enabling Authentication
Next, you need to go and register your application under your Firebase project. On the project’s overview page, select the add app option and pick web as the platform.

Tinder Clone Project Page
Once you’re done registering the application, you’ll be presented with a screen containing your application credentials. Take note of the second script tag as we’ll be using it shortly in our Vue application.
Congratulations, now that you're done with the installations, let's do some configurations.
Installing The CometChat VueJs UI Kit
Copy the folder to your source folder.
Copy all the dependencies from package.json of cometchat-pro-vue-ui-kit into your project's package.json and install them.
Configuring CometChat SDK
Inside your project structure, open the main.js and paste these codes.
The above codes initialize CometChat in your app and set it up. The route guard will filter out unauthenticated users. The main.js entry file uses your CometChat API Credentials. This file also contains the Firebase Configurations stored in the .env file. This .env file will not be public on your git repo as specified in the .gitignore file.
Setting Up The Router
The router.js file has all the routes available in our app along with their security clearance.
Setting Up The Firebase SDK
The firebase.js file has all the codes to interact with the auth firebase service. It will also make our code redundant-free.
Project Structure
The image below reveals the project structure. Make sure you see the folder arrangement before proceeding.

Tinder Clone Project Structure
Now let's replicate the rest of the project components as seen in the image above.
The App Component
The following code wraps around our app within the vue-router enabling smooth navigation. For each route, this component generates a new id to improve the expected behavior of our app.
The Sidebar Component
The sidebar component showcases matched users. Other than its elegant design, it gives users the ability to chat with other matched users. Other things that it does aside from the above mentioned is to provide searching and logout abilities.
The Messages Sub-Component
The Sidebar component contains a child component called "Messages". This child component lists the matched users associated with the currently logged-in user. Here is the code for it.
The Authentication Components
The authentication components include the registration, login, and forget password components. Let make each of those files within the "views" folder and the instruction is as follow.
Make a new folder called “views” and create the following components inside of it. They should all end with the ".vue" extension of course. The Login, Register, and Forget components and must also contain the following codes.
The Register Component
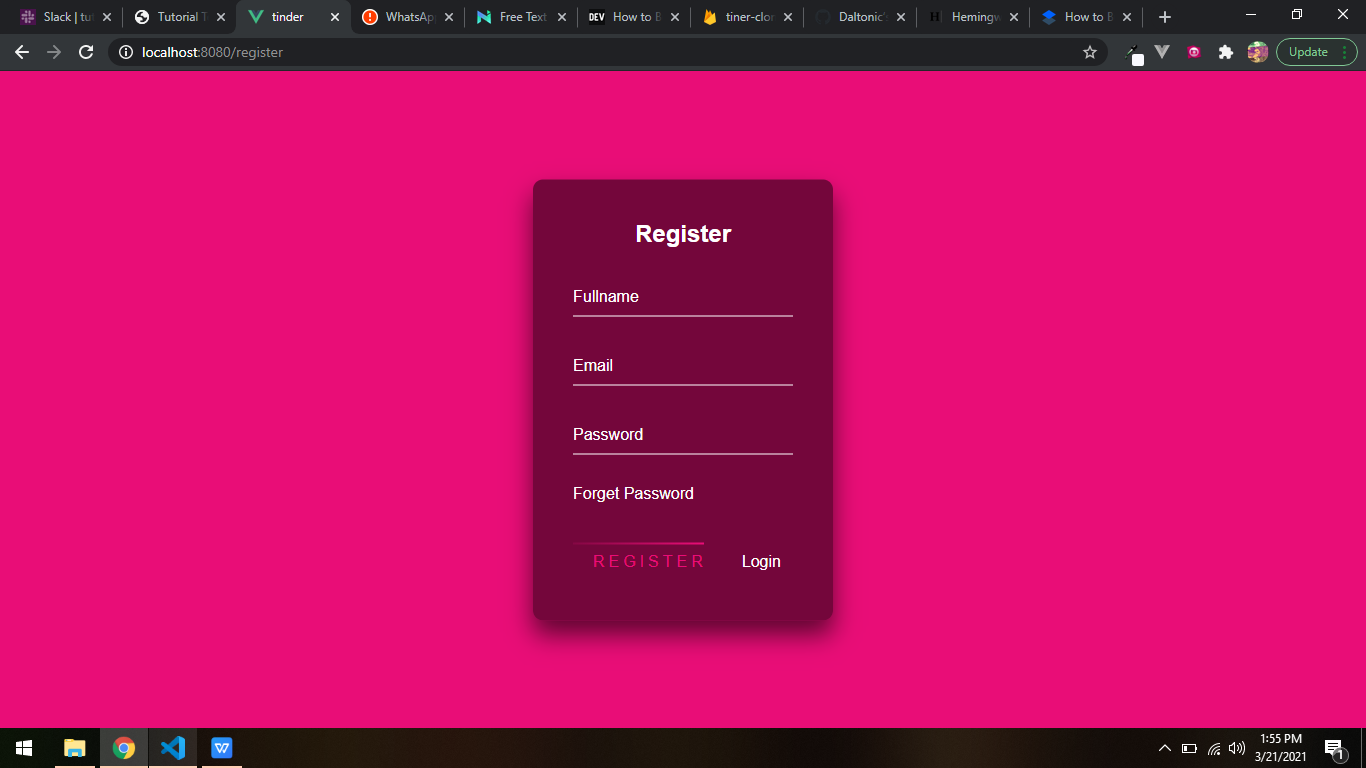
The Registration Screen
We want a situation where a user can click on the "register button" and send his record to Firebase. After firebase registration, the CometChat SDK will also register the new user. This registration will be under the API key you created earlier on.
For instance, a user named Lucy wants to register on our platform. So she enters her credentials within the registration form provided and clicks the register button. Now, firebase sees her details and registers her if her email is unique. After the registration with Firebase, CometChat takes over and also registers her. CometChat uses your API key and places her under your account and her ID is set to her firebase ID.
The script below describes the authentication process in detail. Here is the full code for the registration component.
The Login Component

The Login Screen
Once a user clicks on the Login Button with his detail entered in the Login form, firebase commences validation. If firebase validation is successful, CometChat signs the user in with his firebase ID. This follows the same principle as the registration process.
Below is the full code for the Login component.
The Forget Component

The Forget Password Screen
The forget password component is important for recovering passwords. The Firebase SDK provides that functionality. Also, to make our App complete we have to include it.
The code in this component allows you to recover lost passwords using a valid email address.
The Profile Component

The Profile Component
The profile component is responsible for capturing and updating the rest of a user’s details. Once a user registers in our app, he will be directed to the profile page to complete his details. These details are important to the proper functioning of our application. A user will not be allowed to continue with the system until he completes his profile. The information the profile component requires is as follows.
User’s Fullname
User’s Avatar
User’s Age
User’s Description
Below are the codes responsible for this operation.
Now that we're done with the Authentication components, let's jump into the last three most important components.
The Home Component
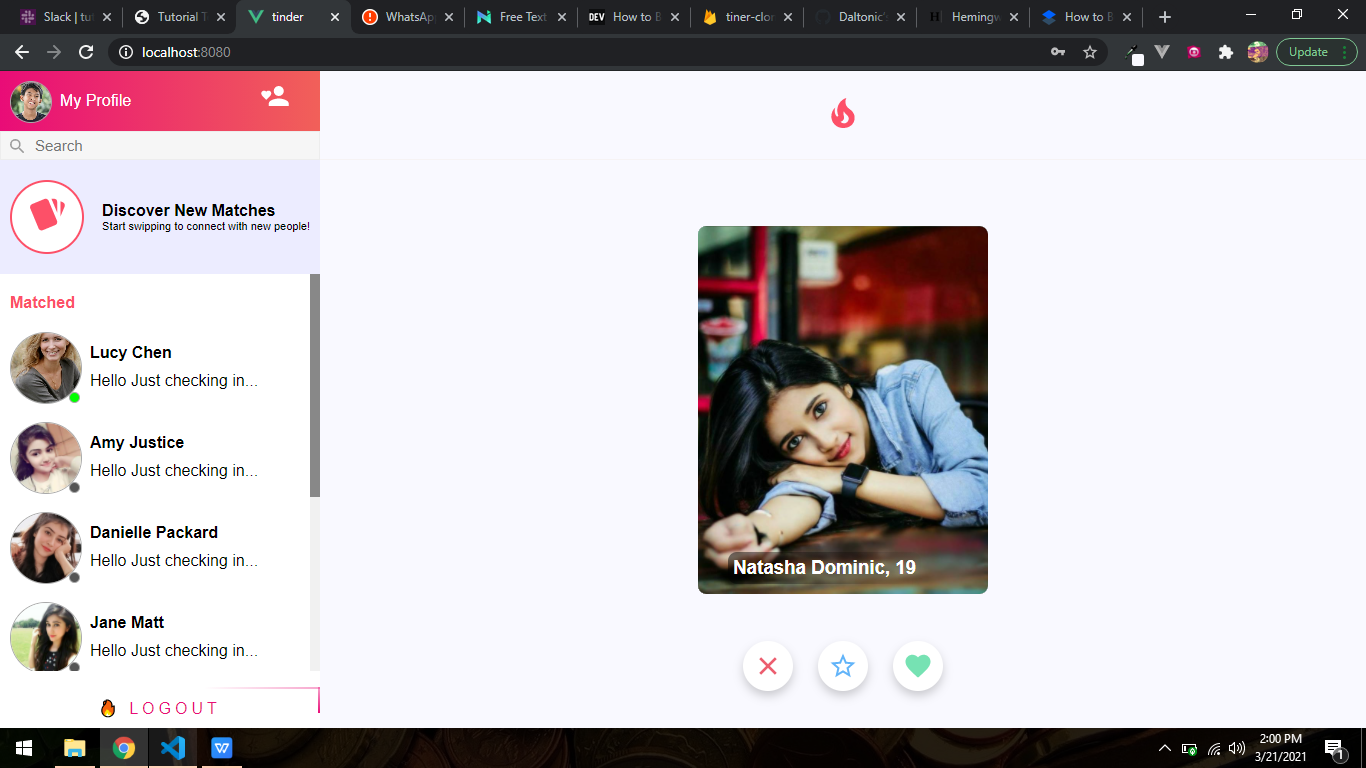
The Home Screen
The Home component carries two child components, the MainHeader and TinderCards components. Other than its beautiful design it also interacts with the CometChat SDK. Here is how it functions.
On create, the Home component retrieves the list of users within our CometChat account. After retrieval, it serves them to the TinderCards child component. The code below illustrates my point better.
Here is the full code of the Home component.
The Child Components
While the MainHeader child component displays the navigation buttons. The TinderCards child component showcases the cards along with the well-styled buttons. Here are their respective codes.
Now that we're done with the Home component and its children, let's talk about the Chats component.
The Chat Component

The Chat Screen
The Chat component lunches a warm and gorgeous chat UI that gives "Tinder.com" a run for its money (smiles). It gives a user the ability to engage in text conversations. Let's look at the code responsible for this functionality.
Let me explain further, there are three methods you should pay close attention to. They include getUser(), getMessages(), sendMessage(), and listenForMessage().
The getUser() method as intuitive as its name sounds retrieves a user from your CometChat account. After the retrieval, it saves the details in a user property for other usages.
The getMessages() method collects all the conversations between you and another user. Afterward, it stores it up in a messages array for further use.
The listenForMessage() method invokes a listener between two users engaged in a chat. It updates the view with the new messages sent by either user.
Lastly, the sendMessage() method sends a text from the one typing the message to the one receiving.
I bet you have got a better understanding of how that process works now, let's move on to the Friends component.
The Friends Component

The Friends Screen (Vue UI Kit)
The Friends component is yet another important component as it carries the full power of CometChat. It contains all the functionality of CometChat. Yes, you heard me right. These functionalities include the CometChat Vue UI Kit, chatting, audio, video calling, and more. Let's see its simple code below.
The Vue UI Kit Customization
Access the following files within the Vue UI Kit component and change them to the following codes.
' .../src/cometchat-pro-vue-ui-kit/src/components/Users/CometChatUserListWithMessages/style.js '
' .../src/cometchat-pro-vue-ui-kit/src/components/Users/CometChatUserList/style.js '
' .../src/cometchat-pro-vue-ui-kit/src/components/Users/CometChatUserList/ CometChatUserList .vue '
' .../src/cometchat-pro-vue-ui-kit/src/components/Messages/CometChatSenderTextMessageBubble/style.js '
' .../src/cometchat-pro-vue-ui-kit/src/components/Messages/CometChatMessageList/style.js '
.../src/cometchat-pro-vue-ui-kit/src/components/Messages/CometChatMessageHeader/CometChatMessageHeader.vue
Conclusion
To conclude, we have covered a step-by-step process on how to build a dating site using tinder as a case study. We've learned how to integrate the CometChat SDK in solving communication problems on the web. We've explored the various functions within the CometChat SDK to send and receive text, audio, and video calls. Now that you've seen how easy it is to use the CometChat SDK and UI Kit, it's time you get your hands on deck and create something else with it.
About Author
Gospel Darlington is a remote full-stack web developer, prolific in VueJs and PHP API development. He takes a huge interest in the development of high-grade and responsive web applications. He is currently exploring new techniques for improving progressive web applications (PWA). Gospel Darlington currently works as a freelancer and spends his free time coaching young people on how to become successful in life. His hobbies include inventing new recipes, book writing, songwriting, and singing.

Gospel Darlington
CometChat