In this tutorial, we will be building a chat application using CometChat and Angular. In the chat app, a user can register/log in and begin chatting with their contacts, also the user can create a group chat and video call their contacts.
The best case study will be WhatsApp, since WhatsApp is a messaging application that lets users text, chat, and share media, including voice messaging and video, with individuals or groups.
So what is CometChat?
CometChat is a robust Chat API & In-App Messaging SDK for Mobile & Web Apps. It readily integrates with over 92+ platforms and comes with inbuilt monetization, moderation, collaboration, and administration tools. CometChat also comes with a Chat UI kit with a fully customizable source code.
Prerequisites
Setting up a Server
To start, we will first create an Express server that will be storing our registration and login data in MongoDB so we can complete authentication later in our Angular application.
For the database, we will be using MongoDB and we will connect it to our server-side (Express) application. To use MongoDB, you will first have to create an account. When you are done creating the account, you can then get the link to your MongoDB database to be stored later in your .env file.
Next, you can go ahead to set up your server-side using express. I have gone ahead to create an express application for the server-side and I won’t document it in this tutorial to shorten it. But you can clone the files on GitHub using this repository link.
When you are done cloning the GitHub repository, you will install the package file required on package.json using npm:
npm install
When the installation is complete, create a .env file to store our environment variables such as PORT, our MONGO_DB link, and an ACCESS_TOKEN_SECRET for JWT. The access token secret can be anything random from your name to a combination like password.
Here is an example of what mine looks like:
And you are good to go on the server side of things.
Let’s start and keep the server running:
npm start
Create Angular Application
To start we first need to create a new instance of Angular using our Angular CLI. To do that, simply open up your terminal and input the below code
ng new whatsapp-clone
When it is done installing the dependencies, you can then step into the directory whatsapp-clone which has just been created, and kickstart your application.
To do that, use the following codes:
cd whatsapp-clone
ng serve
After we are done with creating our angular application, we can then go ahead and set up our CometChat application in the newly installed Angular directory.
Setup CometChat
CometChat offers a customized chatting UI kit for a variety of frameworks including Angular, which cuts out the stress of building the frontend yourself and you can focus on just customizing to your taste.
To make use of the CometChat UI Kit which has been configured for use on angular applications, we need to first install CometChat. To do that, open up a terminal on your angular application directory and run the below code.
npm install @cometchat-pro/chat@2.2.1 --save
Then we import CometChat and configure it in our main.ts file:
To get your appID and region you have to create an account in CometChat and copy the details from the dashboard.
After that is done, we can then get the CometChat UI Kit from this repository and copy it to our src folder in the application.
CometChat UI Kit in src folder
When that is done, we can then install @ctrl/ngx-emoji-mart using:
npm install @ctrl/ngx-emoji-mart
Then add our style files in angular.json to use the built CSS for our UI Kit:
NOTE: For those using Angular 8 and greater, you will have to turn off some tslint configurations for strict typescript codes.
To do that, simply go to your tsconfig.json file and change the boolean declarations for some cases:
And that is it, we can then begin to use CometChat UI Kit in our application.
Authentication
To begin, we create our authentication components and also our layout components to separate the display when a user is logged in or not, we also create our authentication service to communicate with the CometChat and our Express application APIs. After that, we generate a guard which will protect the routes that we don’t want a user that is not logged in to have access to. Let’s dive in.
Layouts
We will create two layout components Default and Blank. The default layout will contain the layouts for routes that have been authenticated, then the blank layouts will contain routes that are not logged in. Let dive in
ng generate component components/layouts/default && ng generate component components/layouts/blank
Then we include them in our app.module.ts file:
Authentication Components
Next, we generate our authentication components which will communicate with our authentication service, and also get data from our HTML pages and fetch data too.
To generate components we will simply use the angular component generate command:
ng generate component components/auth/login && ng generate component/auth/register
Authentication Service
After we are done, generating our authentication components, we can then generate our service and use it to communicate with the CometChat and Express application APIs.
ng generate service services/auth/auth
Then we create functions in our service to communicate with our login and registration APIs.
Let’s break down what is happening above:
We have created a login function that connects to the URL added in our environment.ts file using a post request
The loggedIn function checks a user’s access token from our localStorage which we will be using for authentication in our angular application.
The getToken function gets the accessToken from the database for consumption and guard request verification.
The register function connect with our register API using the post method provided by the httpClient we imported.
For registration on CometChat, we added a function to register our user on CometChat which is the registerCometChat function.
Authentication Guard
With our services setup, the next thing we need to do is add our guard to check if your accessToken is stored and protect the routes if it isn’t.
To start, we will generate our guard in the command terminal:
ng generate guard guard/auth
Then we update our canActivate functionality in the guard file to check for the passed accessToken and then verify the route.
Register
So now we are done with the requirements for authentication, we can now go into creating a registration form and also connecting to our authentication service.
In our register component, we will create functions to access our authentication service and access the registration function for both express and CometChat.
In the above code, the register function takes registerData object as a parameter and sends it to the authentication service we created earlier. Then when the process of registering for our express application is done, the information is then sent to CometChat to complete registration.
When the registration is complete, we can then login our new user using into CometChat using the cometLogin function, which takes the newly created uid as a parameter.
After we are done with the functionality, the next thing to is to create our registration form which will be done in the register.component.html file:
Did a little styling to make it a bit cool. You can get the styles from the repo.
Register page
Login
After the register has been created, we can then go ahead and update our login component to log our user into CometChat and also our application. To begin, we will create a login function to get the information from our form and send it to our authentication service and if successful, we can then login the user.
Then in our form we add our data to the loginData object in our component:
Routing
Now that we are done with the authentication, we can then create our routes to have access to the pages of the components we have created. So in our app-routing.module.ts file, we will add all our necessary routes and after that create our welcome page which will contain our CometChat UI Kit.
We first generate our welcome component using:
ng generate component components/pages/welcome
Then we update the app-routing.module.ts file:
Welcome Component
Now we are done with authentication and routing, we can then add our CometChat UI Kit to the welcome page to begin chatting with our contacts.
To do that, in our welcome component html, add the CometChat UI tag:
After that, we can then add CometChat to app.module.ts
Now we can begin to use our application and chat with users.
Chat Page
Group Chat
To make use of the CometChat group chat you can navigate to the groups section in the navigation and add a new group
Group Chat
Conclusion
In this tutorial, we built a chatting application that utilizes CometChat UI Kit for Angular and allows us to register and use the application to chat with users and also video call. We covered how to set up our angular application, install and use CometChat and also implemented authentication in our angular application to protect access to it.
I hope this tutorial was helpful and also insightful and I can wait to see how you utilize the great possibilities made by CometChat alongside angular. To get the code in this tutorial, you can clone the repository.
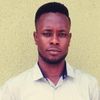
Chimezie Enyinnaya
CometChat