Prerequisites
In order to complete this tutorial and have a working application, some knowledge in the following can be required:
Basic knowledge of React, React hooks and general JavaScript,
Firebase features knowledge,
TailwindCSS, and
Any text editor (I recommend Visual Studio Code)
Introduction
Have you ever been browsing through Amazon trying to find that specific product that you need to follow particular characteristics? You eventually find it, but you have some questions that the product description just doesn’t answer.
How great would it be if you could chat with the seller, on Amazon?!
In this tutorial, you will learn how to create a React App from scratch that will serve as a basic Amazon Clone with chat capabilities right on the product page using some of CometChat’s features and components with easy steps to follow along!
On the product page, you will have a button to start a chat with the seller. If you are the seller, all chatting requests will reach your inbox, where you can help customers resolve their issues.
Perfect!
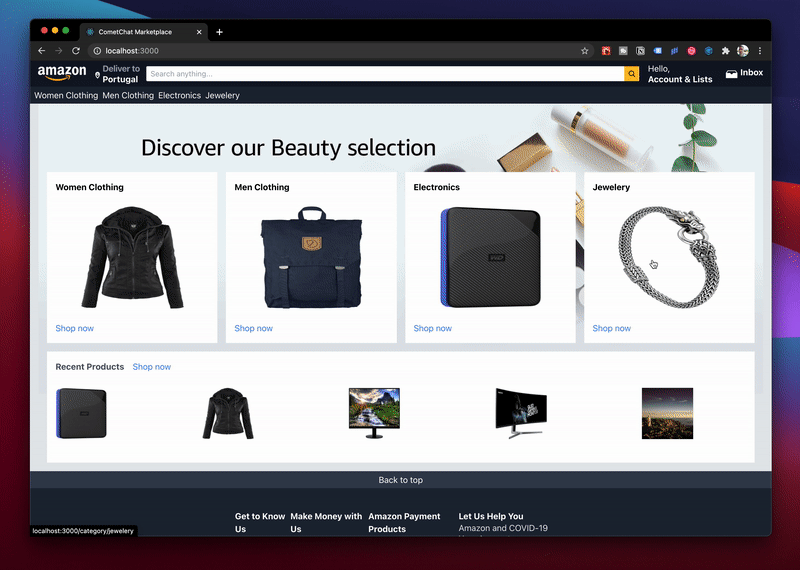
Desktop user interaction and chat
Step 1 - Creating a React App
Create React App
Our first step should be to create the scaffold of our vanilla React app and, for that, I like to use the create-react-app package. So, using npx to not need to have the package installed globally, you can run the following command on the folder you’d like your project to life.
_npx create-react-app chat-marketplace
_
You now have a new folder named chat-marketplace that will hold all of our code and configurations.
Install TailwindCSS
In order to install TailwindCSS that we will be using to style our components, please refer to the most updated official tutorial on TailwindCSS docs related to using the Create React App starter, here.
After the setup, replace your tailwind.config.js with the following, for best results.
Step 2 - Preparing Firebase Integration
The next step you should take in order to get this up and running is to set up your serverless backend. For this project, we are using Firebase to handle our user authentication and to store our application data. A Firebase project was already provided and configured with the environment variables if you copy the .env.example file in the repository and rename it by following the README instructions, but if you choose to create your own, please follow the steps below.
Creating a Firebase Project
If you go to Firebase’s website here, you are able to login with your Google account and create a new project. Name it something like Chat Marketplace. Once you are there, you are now able to add apps to your newly created project. Choose Web App and you are presented with your much necessary project credentials that you will need in order to run this project.

Firebase Project Credentials
On the root of your React project, create a new file .env with the following contents, replacing the values with your own project credentials.
Enabling Authentication
Firebase has the built-in capability of managing users authentication and state. To be able to take advantage of this we must first enable Authentication within our project by going to the sidebar option and enabling Email and Password Signin method.

Enabling Authentication in Firebase
Initializing your Database
For storing data we will be using Firebase’s Firestore database which is a No-SQL database on the cloud. You also find it on your project’s sidebar and go through the configuration process. At the end, you should be presented with an empty database. We now need to add data to it so that our application doesn’t load empty.
You should create two collections, one named categories and another called products. After that, create a few category documents on the first collection adding a name and image attributes to each document of the collection.
For the products collection, each entry should have at least the following attributes: category (should be the name of one of the previously created categories), description, id, image, price, title.

Cloud FIrestore Schema Example
We should now have everything ready regarding our serverless backend and can move on to integrating it with our React project.
For seamless integration, we will be using the React Firebase package. To install it, run the following commands.
npm i firebase && npm i @react-firebase/auth && npm i @react-firebase/firestore
The next step of the configuration is creating a firebase.js file on the src folder of your project where the configuration of your firebase app will be constructed and later used.
Step 3 - Preparing CometChat Integration
Get your keys
As you may know by now, we will be using the amazing real-time chat solutions provided by CometChat.
Let’s start by creating a CometChat account by heading to their signup page. After registering, you will land on your new CometChat dashboard and you can now create an App.

CometChat Dashboard
Once you create your app and select it, you will find the necessary configuration information right at the top of your page. These are the appId, authKey and region.

CometChat App Page
Copy those value and add them in the previously created .env file with the variables names like below:
Install CometChat Dependencies
To install CometChat required dependencies to your React app, run the following command.
_npm install @cometchat-pro/chat@2.2.1 --save
_
That is it.
Create a Helper File
This step is aimed at abstracting most of CometChat’s required steps in order to register, login, creating and joining groups into simple functions that can be called from anywhere within the app.
On the root of your src folder, create a file called cometchat.js and with the following contents.
Copy the React UI Kit
Since we will be building our marketplace app with React, we will be taking advantage of the pre-built CometChat React UI Kit where we are given all the necessary components to make this work, without any further implementations and designs of our own!
To do that, head over to the CometChat React UI Kit repository and clone it. Move the CometChat Workspace folder to your React app src folder and copy the repository’s dependencies to your package.json and run npm install to install them.
Now all configurations are set and we can start coding away our frontend project!
Step 4 - Putting the Pieces Together
Now it is time to combine all of the above and to create the pages that will make our marketplace with real-time chatting capabilities.
Create the entry point
At the root of your src folder, create a index.js with the following:
This code is responsible for initializing CometChat and setting up Firebase and providing it to the rest of the app.
Setting up our router
The next important step is to create our React Router that will enable us to navigate through our pages.
There should be an App.js file on the src directory with the following code:
You now added the capabilities of moving through pages and protecting routes from unauthenticated users by checking if they are logged in prior to rendering the corresponding component!
Create a Layout Wrapper
This is a nice practice I like to have in order to keep code duplication to a minimum, and ease of development. Create a High-Order-Component that you can use to wrap around the render of each page, taking care of common elements like the footer and navigation bar, as well as providing common and necessary props to each page.
On the src directory, create a new wrappers folder and in it, a layout.js file with the following content:
Now, any component can be wrapper with withLayout(Component) and will be rendered within the same conditions.
Creating our Components
On the src directory, create a components folder where all of our components will exist.
Let’s create our navigation bar first. Create a Navbar.js file with this content:
Next, we are creating the footer. The same as before, create a Footer.js file with these contents:
Now our most common components which are part of our Layout component are finished and we can move on to your specific on-page components.
Let’s start with our category cards that feature on the main page. Create a CategoryCard.js and add the following code:
Still for the main page, let’s create a product slider which features horizontal scrolling! Create a ProductSlider.js with the following:
When we are on a specific category page, we have multiple products being displayed on a very structured manner. Let’s create that specific individuallized component, ProductCard. Create that file and add the code:
All that is left, component-wise, is the side-filter that we normally see on Amazon’s product search pages. Ours is entirely mocked with no real function, but it serves it purpose. Add a new SideFilter.js file and paste the contents:
All done now. Let’s create the pages!
Creating the Pages
We already have a handful of components, but nowhere to display them since our router can’t even find the pages it needs to properly manage navigations, so let’s fix that. Still on the src folder, create a new directory to hold our pages. Call it pages.
Starting with Login and Register pages, create two files named login.js and register.js and add the following code to each, respectively.
login.js
register.js
Now going onto the main pages, the index page is the first time we resort to our Firestore collections in order to render it, since it will display both category and product lists! Create a new index.js file and paste its contents:
The rest of the pages are pretty straightforward. We will be creating specific pages for category, product, new product, and a simple logout.
category.js
product.js
newProduct.js
logout.js
Now comes one of the most important pages and yet, the simplest. The CometChat integration for visualization of conversations, for the ability to send and receive messages can be narrowed down to a single line of code using the CometChat React UI Kit. We integrated CometChat into its own page that we called the Inbox page. Create a new inbox.js file and paste this few lines:
And that is it, you now have a fully-fledged chat window!
Step 5 - Seeing the Magic Happen
If everything went according to plan, you can now run the following command to start your web application locally and visit it at localhost:3000:
npm run start
If it compiles the first try, all that is left now is to create some users and get them chatting with one another!
Wrapping Up
Hopefully, with this tutorial, you were able to create a working marketplace web app with real-time chatting capabilities and no backend coding of any kind.
You have configured a fresh Firebase project, inserted No-SQL data into a cloud data source, authenticated users, set up a real-time chat solution with CometChat and got your users sending messages to each other in around 30 minutes or so.
Hope you find this tutorial helpful and don’t forget to check the official documentation of CometChat to explore further and add more features to your app. The complete source code to this tutorial is available on GitHub.
About the Author
Igor is a Fullstack Developer based in the beautiful Oporto city, Portugal. he has a BSc + MSc in Software Engineering but his greatest passion right now is creating online content to help anyone who is trying to get into software development! Apart from coding as a full-time job and being active on Twitter, he writes and maintains dailydev.io. You can also find his articles on Medium, DEV.to and Hashnode.
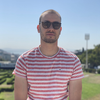
Igor Silveira
CometChat