In this tutorial, you will learn:
1. How to set up a simple one-to-one chat
2. How to add group chat functionality
3. How to configure advanced features like read receipts and presence indicators
Prerequisites
Before you begin, it's important to understand the tools and technologies you will need in order to follow along and have an idea of what you are going to build.
Make sure you have the latest Flutter SDK installed on your system. You'll also need an Android device (physical or emulated) for testing the app on Android and a Mac machine and an iOS device (physical or emulated) for testing the app on iOS.
You'll need to create a trial account in CometChat and create a new app in the CometChat dashboard.
Add real-time chat with minimal effort using CometChat
Once done, retrieve the app ID, auth key, and region from the Credentials page of your newly created CometChat app in the dashboard to use later in development.
This tutorial explains how to build a bare-bones app that allows users to log in with their user ID and view their conversations. From the conversations screen, they will be able to initiate one-to-one and group chats. The following diagram illustrates how the user flow works:
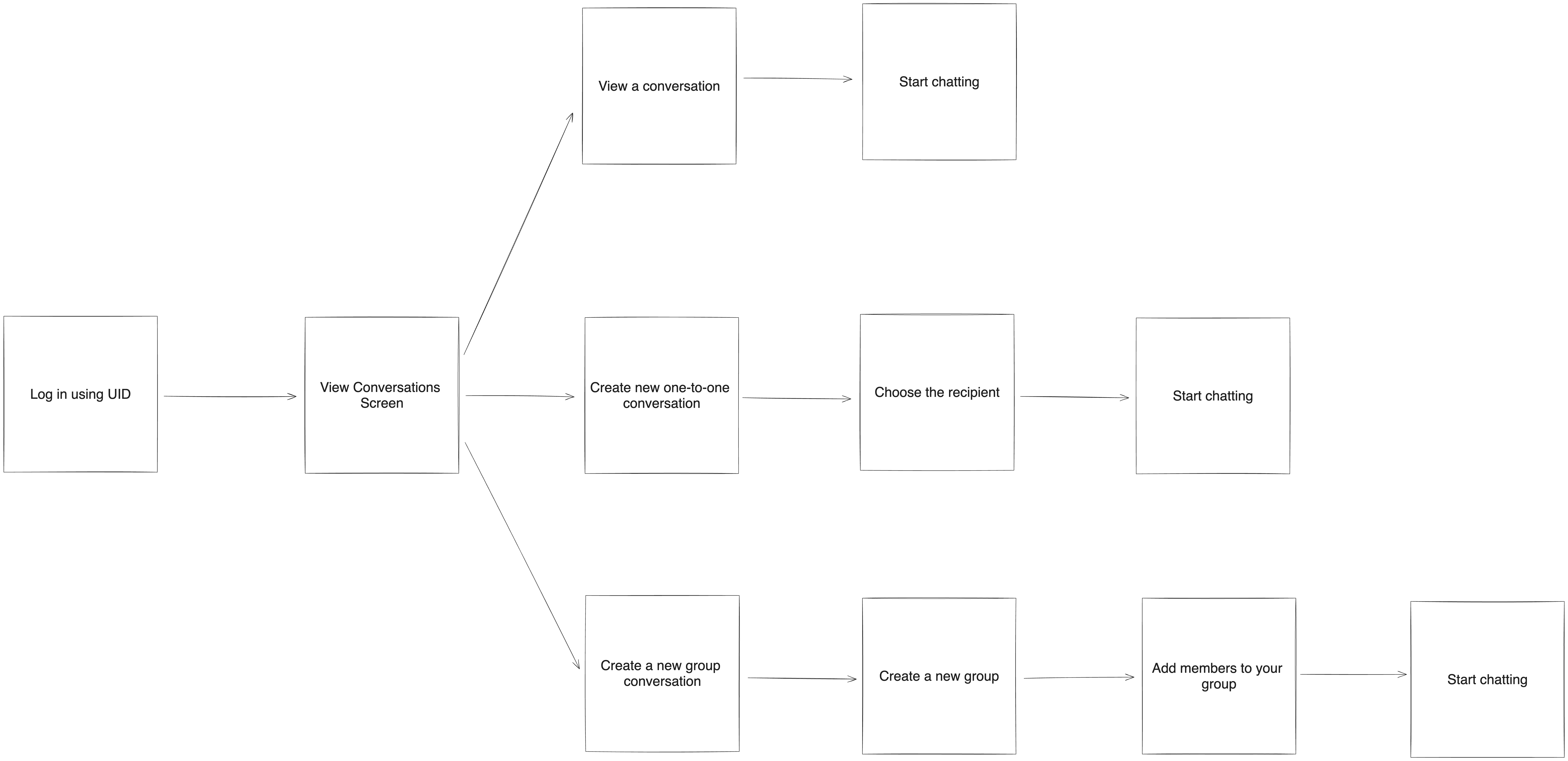
Developing the Chat App
Now that you understand the structure of the app you're going to build, it's time to start developing. Begin by opening up a new terminal window and running the following command in it to create a new Flutter project:
Once the app is created, open it in an IDE of your choice. Navigate to the lib/main.dart
file and replace its contents with the following:
This will set up a basic home screen for your app, where you'll add the CometChat initializations later on.
Setting Up CometChat
The next step is to install and set up the CometChat SDK and UI Kit. To do that, open up your pubspec.yaml
file and add the following line in the dependencies
array:
Run flutter pub get
to install the newly added package. You'll also need to make a few more changes to properly configure CometChat.
For iOS, put the following code at the end of your ios/Podfile
to exclude arm64-i386
architecture from your list of simulator architectures and disable bitcode.
These configurations are needed to work with the CometChat libraries.
Also, add the necessary permissions in your app by adding the following in your info.plist
file:
Make sure to run pod install
in the ios
folder after you add this. Your deployment target should also be set to 12 or higher.
For Android, navigate to android/app/build.gradle
and set your android.defaultConfig.minSdkVersion
to 21 and android.defaultConfig.targetSdkVersion
to 30.
Once done, you're now ready to initialize and use the CometChat SDK and UI Kit. You can add the following import line to the Dart file where you intend to import and use the CometChat UI Kit:
In case you run into any issues up to this point, feel free to check out the official setup documentation from CometChat for help with troubleshooting.
Now, go back to your lib/main.dart
file and replace the initState()
function in the _MyHomePageState
class with the following:
This will initialize the CometChat UI Kit in your Flutter app using the credentials you retrieved earlier from your CometChat dashboard.
Next, add the following code snippet to the _MyHomePageState
class to create a function that enables users to log into their CometChat account using a user ID:
This code snippet uses the CometChatUIKit.login()
and CometChatUIKit.createUser()
functions to first try a login attempt with the given user ID. If it succeeds, it calls a function named _navigateToConversations
to navigate to the conversations screen. If the login attempt fails, it then creates a user and tries logging in again. On a successful login, it will call the same _navigateToConversations
function to navigate to the conversations screen.
Next, define the _navigateToConversations
function in the same class:
This function navigates the user to a screen named Conversations
. This is where you will make use of the CometChat UI Kit to render a list of conversations and the chat windows. It's with nothing that the UI Kits offer a wide range of customization options, including color palette, font, typography, and buttons, so you can tailor your chat windows to your specific requirements.
Before getting to that, finish the main.dart
file by replacing the build()
function of the MyHomePage widget with the following snippet, which renders a basic form on the screen that enables the users to enter their user ID and initiate login:
Here's what the form will look like:
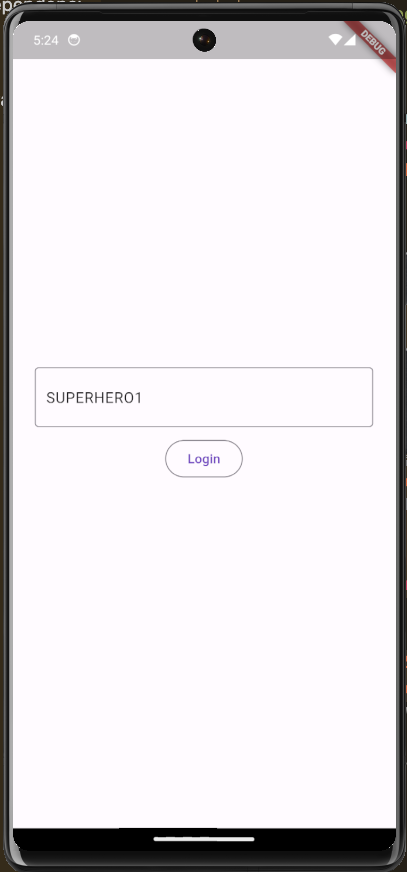
You're now ready to implement the chat functionality in this app.
One-to-One Chatting
To start off, create a new file in the lib
directory with the name conversations.dart
and save the following code in it:
Add the import for this file in the main.dart
file so the user can navigate to it from the app. That's it—this should set up one-to-one chat in your app! All you did was render the CometChatConversationsWithMessages
widget from the CometChat UI Kit, and it will now take care of rendering the complete conversations list with the option to initiate new chats with users and view previous conversations.
You can now run the app using the flutter run
command on two connected devices and try out the one-to-one chat. Feel free to use any of the following five user IDs to start with preconfigured demo accounts: SUPERHERO1
, SUPERHERO2
, SUPERHERO3
, SUPERHERO4
, and SUPERHERO5
.

Group chatting
While CometChat offers a UI component and integrations to help you get started with one-to-one chats quickly, group chats require a few extra steps. To provide you with the most flexibility, CometChat gives you individual components and functions that you can use to build your custom group flow.
The user flow looks like this:
1. The user clicks on the “Create New Group” icon from the conversations page.
2. The user is asked to enter the details of the new group (name and visibility).
3. The user is then asked to choose the members of the group.
4. The group is created and all the chosen members are added to it.
Updating the Conversations List Page
To start off, replace the return
statement of the Conversation
class's build
function in the lib/conversation.dart
file with the following code:
This code snippet passes in a few properties to the CometChatConversationsWithMessages
widget to customize it. A ConversationsConfiguration
object is used to set the title of the page, hide the back button, and customize the page's app bar to show two icons: one for creating a new one-to-one chat and the other for creating a new group chat. You can learn about more changes you can make to the configuration here.
You'll need to define the createNewChat
and createNewGroup
functions to kick off the new one-to-one chat and new group chat flows from the main conversations list page.
Navigating to the New One-to-One Chat from the Conversations Page
The implementation of createNewChat
is simple. It navigates the user to the CometChatUsersWithMessages
widget, which shows a list of the users that the logged-in user can choose for one-to-one chatting. To implement this, add the following function to the build
function:
Navigating to the New Group Page from the Conversations Page
The implementation of createNewGroup
needs to be configured manually. The CometChat UI Kit offers two prebuilt components that will help you in this case: CometChatCreateGroup
for entering group details and creating the group, and CometChatUsers
for viewing users to add to the group.
You could simply navigate the user to the CometChatCreateGroup
widget, which will ask them to choose the type of group to be created (public, password, or private), enter a name, and create it with just the logged-in user as the sole member. You can then come back and add more members through the group's settings. However, a more intuitive workflow would be to pull up the list of users after collecting input for group details and ask the user to choose a few users that they would like to immediately add to the group.
To implement this, add a new function called createNewGroup
in the build
function of the Conversations
class with the following declaration:
This function starts off by navigating the user to CometChatCreateGroup
to enter group details as soon as they press the new group button on the conversations page. However, you don't want CometChatCreateGroup
to immediately create a new group when the create group button is pressed, so you need to override its default implementation by passing in a custom onCreateTap
function to the widget.
In this onCreateTap
function, you'll navigate the user to CometChatUsers
and enable multiple users to be selected. Once selected, you will write the logic for creating the group and adding the selected members to it using the CometChat SDK. Here's the implementation for the onCreateTap
function:
As mentioned, the CometChatUsers
widget is triggered when the button to create a group is pressed, specifically if selections are activated and set to allow multiple choices. The widget includes an onSelection
function, which creates a group using the CometChat.createGroup
and CometChat.addMembersToGroup
functions from the CometChat SDK. After the group is successfully created, all recently opened widgets are closed, and the user is directed to the chat page of the newly created group.
You can now run the Flutter app once again to view how the create group functionality works:
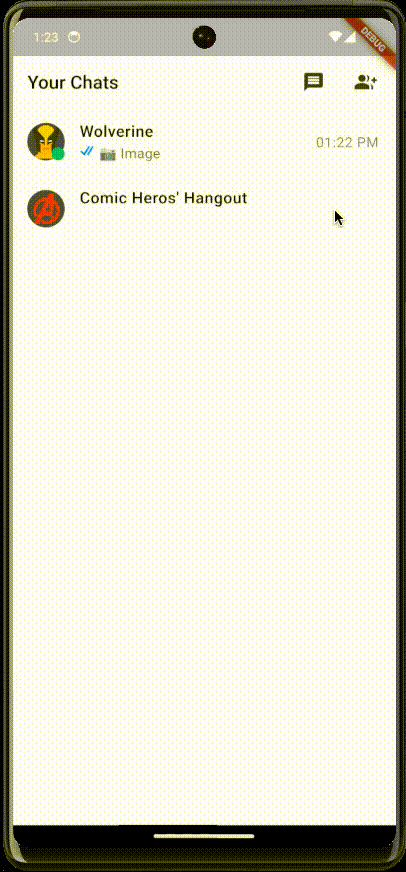
Similar to one-to-one chats, most functionality for group chats is already implemented. You can send and receive text and media messages out of the box and experiment with some advanced features like typing indicators and presence indicators as well. You'll learn more about these next.
Features to Enrich User Experience
Apart from implementing basic chat functionality, you can add some useful features (such as online presence indicators and read receipts) to offer a better experience and engage your users. Fortunately, CometChat offers support for many features that can help you provide a rich user experience.
Presence Indicators
Presence indicators let other users know when you're online or available to talk. The default CometChatConversationsWithMessages
widget enables presence indicators out of the box, so you'll see which users are online in your contacts straight from the conversations list:
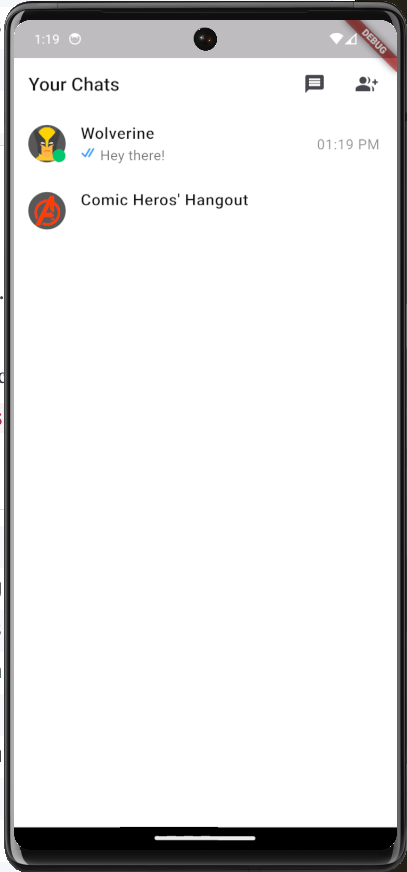
You can disable this feature by passing in an option to the widget
The UI Kit also allows you to customize how the indicator looks by passing the statusIndicatorStyle
option in the configuration object.
You can also control the visibility of presence indicators in the chat screen by passing in a MessageConfiguration
object to the CometChatConversationsWithMessages
widget, like this:
If you want more granular control over presence indicators (such as being able to view only your friends' presence indicators or only show your presence to those people who share their presence with you), you should check out the user presence setup documentation.
Typing Status
Another engaging aspect of real-time chat is knowing when people are typing. CometChat also supports this out of the box, and this feature is enabled in the default CometChatConversationsWithMessages
widget:

You can disable it by passing in an option to the widget:
You can customize the text that's displayed in the place of <user> is typing...
using the typingIndicatorText
option.
You can also control the visibility of typing indicators inside individual chat screens using the messageConfiguration
option in the CometChatConversationsWithMessages
widget:
Read Receipts
Read receipts are visual elements that let users know if recipients have read their messages or not. They're usually designed as a group of check icons that go from one check (sent) to two checks (delivered) and change their colors (to denote read or unread).
CometChat provides this feature out of the box, and it's enabled by default in the CometChatConversationsWithMessages
widget:
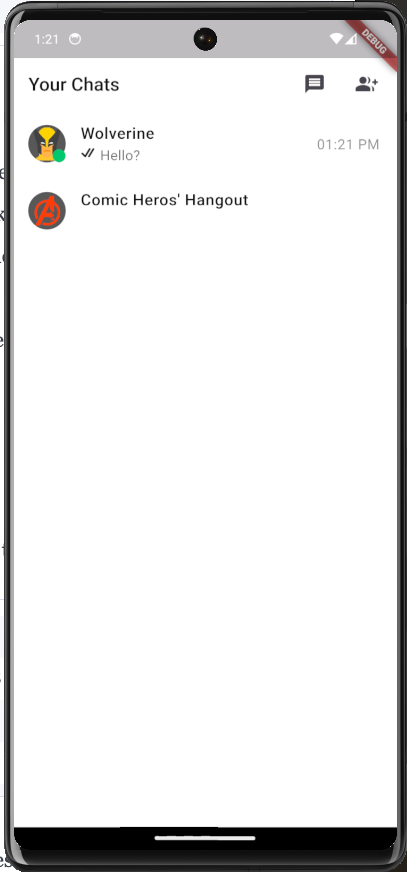
If you want to disable this feature, you can pass in an option to the widget:
CometChat allows you to customize the icon for read messages using the readIcon
option.
You can customize how read receipts look and work inside chats using the messageConfiguration
option in the CometChatConversationsWithMessages
widget:
File Attachments
Supporting file attachments is important in in-app chats since it allows users to share their experiences via images, videos, and audio. Whether it's sharing a fun moment with a friend or contacting in-app support to describe an issue, file attachments always come in handy.
CometChat supports file attachments out of the box in its default CometChatConversationsWithMessages
widget:

File attachments are a part of the conversation only, so you can't customize them directly through conversationsConfiguration
properties. Here's how you can use the messageConfiguration
option to customize the file attachments feature in your app:
Notifications
Notifications are an integral part of any chat application. Without push notifications, it becomes quite difficult to connect with contacts when the app is not open. In most cases, providers such as Firebase Cloud Messaging and Apple Push Notification service are used to deliver notifications across the web. CometChat supports these providers, and you can follow this detailed guide to set up notifications in your app.
Automatic Group Creation
Apart from the standard chat functionalities you saw above, you can also set up custom features such as automatic group creation through CometChat’s UI Kit and SDKs. Automatic group creation can help in cases such as healthcare where doctors, support staff, and patients need to be added to the same group without requiring manual efforts every time.
You can use the create group and add members to a group features of the CometChat SDK to create groups automatically based on business logic and add people to them.
Advanced Chat Features
While the features listed above are sufficient to build an engaging in-app chat experience, you can take it up a notch by adding some advanced features like content moderation and audio/video capabilities.
Moderation
As your app starts to grow, you'll need some form of content moderation to ensure a safe experience for all users. Content moderation refers to the act of ensuring that all content submitted by users to your application (in the form of text messages, attachments, stickers, and so on) adheres to your app's usage guidelines.
This is important since most users look to your usage guidelines to know what kind of experience to expect. Nobody would like to receive NSFW content in a professional app or sales spam in a community app. This is why it's important to set up moderation once your user base grows.
CometChat offers various ways to moderate content through its platform. For starters, you can set up data masking for sensitive information and filters to weed out profane content automatically.
You can also allow users to report other users and their messages. If you're looking for something far more serious, you can consider setting up sentiment analysis or even in-flight manual message moderation to ensure that not a single piece of inappropriate content passes through your app's chats.
The UI kits also support moderation, data masking, and profanity filtering as extensions, which you can learn more about here.
Video Calling and Audio Calling
The ability to initiate video and audio calls is a popular add-on for most messaging experiences today. These calls allow you to connect with your contacts in a seamless, real-time experience. If you're looking to host webinars or other online events, you can make use of these to set up group calls easily.
CometChat offers dedicated support for setting up video and audio calling functionality in your app. With features like whiteboards, documents, and screen sharing, you can build a full-fledged video conferencing app of your own using CometChat.
Conclusion
In this tutorial, you learned how to integrate real-time flutter chat in your app using build real-time using CometChat's offerings.
From understanding the fundamentals of in-app chat functionality to implementing a seamless chat interface, you should now have the required skills to transform your Flutter applications into interactive hubs for meaningful communication.
You can refer to the complete code of the demo app built in this tutorial in this GitHub repository
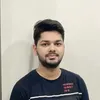
Kumar Harsh