This tutorial is outdated. We're currently working on an updated version. In the meantime, for instructions on building a chat app using CometChat, please visit our documentation.
App and web development have come a long way over the last few years. We use a lot of voice and video chat app every day, including Messenger, WhatsApp, Snapchat and so on. One of the most widely used features is voice and video chat.
Using the CometChat's Android video calling SDK, Firebase backend services, you will learn how to build a voice and video chat app with minimal effort.
Follow along the steps to build a voice and video chat app that will allow you to do the following things
User management
1. A way for end-users to signup(email & password is sufficient)
2. A way for users to log in and have a short profile (Name, UID, Photo, About)
Chat management
1. Use our Java UI Kit and configure it such that-
List of Users/Contacts is visible to all users with a search bar
All users can initiate voice & video calls with individuals and groups
Users can create/exit groups and add/remove other users
Group chat via text, voice, and video must be enabled for all users
2. Login the logged-in user to CometChat
3. Add API call when a user registers so that the user is created in CometChat
Prerequisites
To follow this tutorial, you must have a degree of understanding of the general use of Android. In this project, we will use Android Studio to build the Android app. This will help you to improve your understanding of this tutorial.
Installing the App Dependencies
Step 1: you need to have Java - JDK 8 and Android Studio installed on your machine
Step 2: create a new project with the name voice-video-chat-app by using Android Studio.
Step 3: Replace your dependencies inside build.gradle file (app level) with the following part.
Step 4: Add the repository URL to the project level build.gradle file in the repositories block under the all projects section
Note
If you are using Android Studio Arctic Fox 2020.3.1, the repository URL should be included in the settings.gradle file not the build.gradle file (project level).
Step 5: Inside your build.gradle file (app level), please add the following section.
Step 6: Sync the project.
Configuring CometChat SDK
Head to CometChat Dashboard and create an account. Register a new CometChat account if you do not have one
2. After registering a new account, you need to the log in to the CometChat dashboard.
3. From the dashboard, add a new app called "voice-video-chat-app".
4. Select this newly added app from the list.
5. From the Quick Start, copy the APP_ID, REGION, and AUTH_KEY, which will be used later.
6. Navigate to the Users tab, and delete all the default users (very important).
7. Navigate to the Groups tab and delete all the default groups (very important).
8. Create a file called Constants.java in the package folder of your project.
9. Import and inject your secret keys in the Constants.java file containing your CometChat and Firebase in this manner.
10. Make sure to include the Constants.java file in your gitIgnore file from being exposed online.
Setting Up Firebase Project
According to the requirements of the voice and video chat application, you need to let users create a new account and login to the application, Firebase has to be used. Head to Firebase to create a new project and activate the email and password authentication service. This is how you do it:
To begin using Firebase, you’ll need a Gmail account. Head over to Firebase and create a new project.
Firebase provides support for authentication using different providers. For example, Social Auth, phone numbers, as well as the standard email and password method. Since you will be using the email and password authentication method in this tutorial, you need to enable this method for the project you created in Firebase, as it is by default disabled.
Under the authentication tab for your project, click the sign-in method and you should see a list of providers currently supported by Firebase.
Next, click the edit icon on the email/password provider and enable it.
Now, you need to go enable Firebase Realtime Database. We will use Firebase Realtime Database to store the information of the users in the application. Please refer to the following part for more information.
Choose “Realtime Database” option
Click on “Create Database"
Select location where you realtime database will be stored
Select “Start in test mode” for the learning purpose
Please follow the guidance from Firebase. After following all steps, you will see the database URL. If you just need to update the “FIREBASE_REALTIME_DATABASE_URL” variable in your “Constants.java” file with that value.
On the other hand, your Firebase real-time database will be expired in the future. To update the rules you just need to select the “Rules” tab and update the date/time in milliseconds as you can see in the image below.
The below images demonstrate the data structure of the application. A user should have an avatar, an email, an id.
To set up Firebase for the Android app, we can use Firebase Assistance. You need to follow the below steps to enable Firebase Assistance inside your Android Studio.
Step 1: Click Tools and then choose “Firebase”.
Step 2: Open the assistance by click on the “Assistance” on the right of your Android Studio.
Step 3: You can expand “Authentication” part and “Realtime Database” part and follow the tutorials from Firebase Assistance.
Configuring Strings, Styling, and Themes for the App
Inside our voice and video chat app project structure, you need to follow the below steps to create strings, styling and themes with the below steps, by doing this, we can save time and speed up the development process. We will focus on the business logic in the following section.
Copy the values from here and then paste to your “strings.xml” file.
Copy the values from here and then paste to your “colors.xml” file.
Copy the values from here and then paste to your “themes.xml” file.
The Login Page
This component is responsible for authenticating our users using the Firebase google authentication service. It accepts the user credentials and either signs him up or in, depending on if he is new to our application. See the code below and observe how our app interacts with Firebase and the CometChat SDK.
The image describes that the user needs to input the email and password. To log in to the application, the user needs to click on the “Login” button. The application will validate the user’s credentials first and then call Firebase Authentication Service. If everything is fine, the application will also let the user log in to the CometChat as well. The details about those parts will be discussed in the following parts of this section.
The UI XML code can be found here and the code logic for the Login Activity can be found here. You can refer to the below code snippet for more information.
As mentioned above, if the user’s credentials are valid, we will call the Firebase Authentication service and the CometChat service. Moreover, we need to initialize CometChat in our application and we also call the “loginCometChat” function, this function will take responsibility for letting the user access the CometChat. You can refer to the below code snippet for more information.
According the the UI, the user can go to the sign up page to create a new account by clicking on the “Register” text. The sign up page will be discussed in the following section.
The Sign Up Page
According to the requirements, the application needs to provide a way to let the users create new accounts. For this reason, the sign-up page needs to be created to achieve that. From the login page, after the user clicks on the “Register” text, the user will be redirected to the sign-up page. As you can see on the beside image, the user needs to input some information to create a new account such as the user’s name, user’s email, user’s password, and confirm password.
The last but not least, to create a new account, the final step is to click on the “Register” button. After clicking the button, the application will register a new account by using the Firebase Authentication service, insert new user’s information to the Firebase Realtime Database and then register a new account on CometChat.
The UI XML code can be found here. And the code logic for the SignUpActivity can be found here. You can refer to the below code snippet for more information.
What we have done in the “SignUpActivity.java” will be similar to the “LoginActivity.java”. After the user inputs enough information and then clicks on the “Register” button, the “registerCometChatAccount” will be dispatched. You can refer to the below code snippet for more information.
The following section will demonstrate how to integrate CometChat Android UI Kit to achieve text chat, voice, and video calling features in our application.
Integrate CometChat Android UI Kit
To integrate CometChat Android UI Kit, you need to follow the below steps:
Step 1: Clone the UI kit from android-chat-ui-kit-repository.
Step 2: Import uikit Module from Module Settings.( To know how to import uikit as Module visit this link),
Step 3: Re-check your folder structure. If the library is added successfully it will look like mentioned in the beside image.
Step 4: Add the following parts to your build.gradle file (app level).
After integrating CometChat Android UI Kit successfully, you need to use the following statement to open the CometChat UI Screen. CometChatUI is a way to launch a fully working chat application using the UI Kit .In UI Unified all the UI Screens and UI Components working together to give the full experience of a chat application with minimal coding effort.
Your UI will look like the following image:
Wrapping Up
In conclusion, we have done an amazing job in developing a voice and video chat application by leveraging Android, Firebase, and CometChat. You’ve been introduced to the chemistry behind the voice and video chat application and how the CometChat Android UI Kit makes chat applications buildable.
You have seen how to integrate most of the CometChat functionalities such as texting and real-time messaging. I hope you enjoyed this tutorial and that you were able to successfully build the voice and video chat app. It's time to get busy and build other related applications with the skills you have gotten from this tutorial. You can start building your chat app for free by signing up to the cometchat dashboard here.
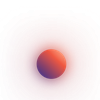
Hiep Le
CometChat