This tutorial is outdated. We're currently working on an updated version. In the meantime, for instructions on building a chat app using CometChat, please visit our documentation.
Live chat is a customer support method with a proven record. It’s fast and efficient since one agent can help many customers at once. Best of all, the quicker you can answer a customer’s questions during the buying process, the more likely that person is to buy.
So, how do you integrate a live chat into your React application?
In this tutorial, I’m going to show you how to integrate a live chat feature into your React app without the worry of maintaining your own chat server and architecture.
Here's a preview of what we’ll be building:
To power our chat application, we’ll be using CometChat Pro.
CometChat Pro is a powerful communication API that enables you to add chat features to your application. With easy integrations and clear documentation, you’ll be able to add a live chat feature into your application with just a few lines of code, as you will soon see. If you want to follow along, you can create a free account here.
Prerequisites
Before we dive into the main functionality, there are some things you will be needing to get started
Setting up a CometChat account and creating an App
React application setup
Creating an agent in CometChat
Creating chat widgets for user and agent
Once you have that then you are ready to start.
Setting up the React app
Inside your directory, run npx create-react-app to scaffold a new React application:
Your folder structure should look like this:
With your React application in place, navigate to the client directory install the following modules:
Creating a React app is really useful to bootstrap a React app, but it also generates a lot of files we don’t need (test files, and so on).
Before we jump into the code, remove everything in the client/src directory except index.js, index.css, App.js .
Update the index.js file as below:
To begin, create a .env.local file in the root directory with your app ID, Region, AUTH KEY, and agent UID inside:
Next, go to the index.html file in the public folder and import the scripts like below in the <head> tag.
Creating the Agent User
Because we will likely have many customers, for each customer who connects to our chat, we will need to dynamically create a CometChat user. However, because there will only be one agent, we can create an "agent" user in advance from the dashboard with any uid and update the .env.local file’s key value.
Creating Chat Widgets for User and Agent
CometChat provides us with inbuilt chat widgets which you can use in your app without any third party libraries. The Chat Widget simplifies the process of integrating a Chat on your website or mobile apps.
As a developer, you only need to toggle the widget's settings from our Dashboard and customize it to fit your needs. Within a few minutes, you can get started with our Chat widget.
The Chat Widget is available for the following platforms:
1. Android
2. iOS
3. Web
4. WordPress
Go to Chat widgets section in the dashboard of CometChat.
Creating the User Widget
Create a new widget for user, this widget will be visible to the new users who come to the website. Add the widget ID from the right sidebar in the dashboard in the .env.local file for REACT_APP_W1 key.
Click on customize from the right sidebar and disable all options except the above one.
Creating the Agent Widget
Create a new widget for agent, this widget is for the agent, who can see all messages and can reply. Add the widget ID from the right sidebar in the dashboard in the .env.local file for REACT_APP_W2 key.
Keep the below settings for agent widget,
Creating the Client Component
Our client component will have two main responsibilities:
Create a new CometChat user when a customer first connects
Send and receive messages in real-time.
Create a file called Client.js inside the src folder and paste the following:
Now, let’s understand the above code,
First, we are initializing the CometChat Widget. While it’s loading we show a spinner to user and after that, we are checking for a user in the local storage. If there is a user inside the local storage we are logging in him automatically, else we create a new user and log him in and store his UID inside the local storage. This way we can preserve the user on page refresh.
Once there is a user logged in, we are simply initializing the CometChat widget with the widget 1 ID that we created earlier and we are starting a chat session directly with the agent we created earlier.
Here is what the application should look like at this point:
That’s it for Client.js file, now let’s see build an agent dashboard.
Creating the Agent Component
The main function of the agent dashboard is to grab all customers from CometChat Pro and display any incoming message from new customer into the customer list chat for agents to click on and reply. The core functionality is very similar to the client.
With CometChat, you could easily create multiple agents but to keep things simple and avoid user management, we have only one agent, which we created earlier.
Create a component called Agent.js inside the src folder and paste the below code:
Now, let's understand the code. We are simply initializing the CometChat Widget and logging in the agent that we created earlier. This time we are going to use the embed view of CometChat Widget. Once the agent is logged in, we are displaying all the list of chats that he received. The agent now can reply to all the messages he receives.
Finally, import the two components in the App.js file and two routes. The file will look like below:
Running the App
Now to start the app, run the below command.
If you have any trouble, you can reference the source code here on GitHub.
Check out the final product:
**Conclusion
**
We’ve made our very own live chat widget for React application, and it took no time at all! Indeed, CometChat Pro enables you to send and receive message by only writing a few lines of code. You don’t need to deal with creating your own chat server and architecture. It also has more capabilities than just making a chat widget.
About the author
A passionate student developer, ReactJS lover, and Javascript enthusiast who also has vivid interest in Web, Android, and Azure Cloud. Know more about Manitej at manitej.rocks!
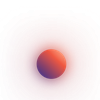
Manitej Pratha
CometChat