This tutorial is outdated. We're currently working on an updated version. In the meantime, for instructions on building a chat app using CometChat, please visit our documentation.
Over the years technology has advanced in several ways and keeps getting better as the years go by. It has been quite resourceful and helpful with daily human activities and other sectors such as online retailing or what is mostly regarded as online shopping. The online purchasing market popularly known as E-commerce is one of the biggest growing industries that has revolutionized online retailing and doesn’t seem to be fading away anytime soon.
In this tutorial, we will build a marketplace site with an important feature to ensure smooth conversation in the form of chat from the prospective buyers of an item and the seller in real time. The application that will be built here will use Laravel for backend logic implementation and Vue.js to handle all frontend interactivity.
Prerequisites
Basic knowledge of building RESTful APIs using Laravel will help you get the best out of this tutorial. Also, ensure that you have:
Composer installed globally on your computer
Application Overview
As mentioned, we will build a market place (E-commerce) platform similar to what you would find on Etsy with chat functionality. The focus of this tutorial is to show you how to easily ensure communication between buyers and sellers of goods. Here is what the goods listing page will look like once we are done:

Once you click on a single good, the image below depicts what the view looks like:

If the end-user is the seller who added the goods, he or she will see a button that will redirect to the inbox page or conversation list once clicked. Otherwise, a message to meet the seller and start a chat will be displayed instead.
Below is what the chat box for each good looks like:

And lastly, a conversation list or inbox page looks like this:

With CometChat infrastructure we will setup and create all the functionalities mentioned above in no time. Let’s get started.
Clone an Application Starter
To get started easily, you are going to clone the starter project in this repository. This starter project already contains a Laravel application with the following:
A Laravel Eloquent model for User
A Migration file to create a Users table
Authentication scaffolding using the Laravel UI package.
Vue.js and its dependencies
To download the starter template, issue the following command from the terminal of your machine:
git clone https://github.com/yemiwebby/lara-market-place-starter.git lara-market-place
The preceding command will install the starter project in a new folder named lara-market-place. Move into the new project:
cd lara-market-place
and install all the dependencies for Laravel using composer by running the following command:
composer install
followed by the command below:
npm install
This will install Vue.js and its dependencies and other JavaScript dependencies.
Setup Database Connection
Next, create a .env file and then copy the content of .env.example into it:
_cp .env.example .env
_
Generate a key for your project using the artisan command:
php artisan key:generate
Now create a connection to your database by updating the values of the properties below within your .env file
Run the application with php artisan serve just to be sure that everything is intact. You will see the welcome page

Create Product Model and Migration
Here, we will create an eloquent model and a database migration file to create Product table and a controller file to manage other product-related activities. Issue the following command from the terminal to achieve all these:
php artisan make:model Product -mc
With the command above we generated the required files as mentioned. Next, you need to make some changes to the default contents of both the migration file and model. Open the Product ‘s migration file in the database/migrations folder and use the following content for it:
You can now update the $fillable property by using the content below for the newly created model in app/Models/Product.php file:
Now, use the command below to run the migration files and create the appropriate fields in the database:
php artisan migrate
Complete the Authentication Process
The starter template already contains both Register and Login controller for authentication. In this section, we will modify the content of both files. Within the RegisterController, we want to be able create a user on CometChat once they register in our application. For this, open the RegisterController found in app/Http/Controllers/Auth folder and update its content as shown here:
In the file above, we specified the required fields that need to be validated during the registration process. And once the user was registered successfully, we made an API call to CometChat’s REST API to create credentials for the user on CometChat as defined in the createUserOnCometChat() method above. Lastly, we generated an authToken to uniquely identify a user on CometChat and save the token against the details in the database.
Login Controller
Next, open the LoginController.php file located in the app/Http/Controllers/Auth folder and use the following code for it:
Once a user has been authenticated successfully, we returned a JSON response with the user’s details.
The Logic for Managing Products
Earlier, we created a ProductController.php file to manage products or goods operations. We want to be able to create goods, display the list of goods or products on a listing page and display a single good. Please note that we want Laravel to return the required views to render the form for creating products and use Vue.js components to handle its logic instead of blade template engine. To begin, start by replacing the content of app/Http/ProductController.php file with the following code:
From the snippet above, we created methods to create a new product, view the lists of products, view a single product and also return a view to render the HTML form for creating a new product.
Update Home Controller
As earlier mentioned, a seller will be able to view the list of conversations started by his or her prospective buyers and respond to them accordingly. For this, open the HomeController.php within app/Http/Controllers folder and include the following to render the inbox view that we will create later in this tutorial:
Update Routes
With all the backend logics out of the way, navigate to routes/web.php file and update its content with the following:
We included the endpoints to reference all the methods created within all our Controllers. These endpoints will be called from within the frontend of the application.
Configure and Set Up Frontend
In this section, we will focus on the frontend logic of the application. This will include creating the appropriate Vue components to consume the backend resources created with Laravel earlier. Also, it is important to note that Vue components will also be used within the blade file created for rendering views. This way, Laravel will still be responsible for routing but when it comes to handling all the logic with the blade template engine, Vuejs components will be used.
Also, we will install and leverage the UI kit created by CometChat to easily configure the chat views. Though we have installed Vue.js and its dependencies, we will begin this section by installing the CometChat javaScript SDK. Run the command below for that purpose:
npm install @cometchat-pro/chat --save
Once the installation is complete, navigate back into the Laravel application and add the following variables to the .env file:
Replace the placeholders with the appropriate details obtained from your CometChat dashboard. Please be sure to use the value of your REST API Key instead of the Auth Key.
Initialize CometChat App
Ensure proper communication between our application and CometChat APIs by initializing CometChat. Open the resources/js/app.js file and use the following code for it:
Creating views and cloning the UI Kit for Vue
To begin, create a folder named views within resources/js folder and create the following files within the newly created folder:
Register.vue
Login.vue
CreateProduct.vue
Inbox.vue
Product.vue
Products.vue
Lastly, we will use the UI Kit created by CometChat team to set up group chat view. This comes with the benefit of an appealing user interface and a proper structure for chat applications. To use the kit, clone or download it from this repository using the following command:
git clone https://github.com/cometchat-pro/cometchat-pro-vue-ui-kit.git
Once you are done, open it with a code editor or any file explorer, copy the cometchat-pro-vue-ui-kit folder and paste it in resources/js folder. Now copy all the dependencies from package.json of cometchat-pro-vue-ui-kit into your project's package.json and run npm install to install them.
Register View
Now, we are set to start creating Vuejs components for all the views in this application. Start by opening the Register.vue file and use the following content to replace its <template></template> section:
Here, we built the HTML form with input fields for user’s name, email, and password. Next, paste the following code after the <template></template> section:
As mentioned, the authentication flow for this application is to register users within it and also create such user immediately on CometChat using the Create User REST API. Here, we created the registerAppUser() to register users within our application.
Login View
Open the Login.vue file and use the following code for its <template></template> section:
We created the input fields for email and password. Next paste the following <script></script> that contains the logic to login a user:
Once a user can log in successfully into our application, we will retrieve the token generated earlier and use it to authenticate such user on CometChat.
Add a new Product
To add a new product, a user must provide the product’s name, description, quantity and price. Populate the createProduct.vue file with the following code to receive those inputs:
Next, add the following to the <script></script> section to include some logic:
The createProduct() method is used to create or add a new product made an API call to the backend resource created earlier. Once successful, we will redirect the user to the homepage where all created products will be listed.
Products Listing
To display the list of products created within the application, open Products.vue file and use the following code for it:
The content above will render a list of products with its details. Next, paste the following code in the <script></script> section of the file:
Once the component was ready, we sent a GET HTTP request to our API to retrieve the list of products from the database.
Lastly, for styling purpose, add the following styles to the <style></style> section of the Products.vue file:
Display a Single Product and Chat With Seller
The single product component will be used to render and view the details of a single product and also render the chat view. Configure this by using the following code for the <template></template> section of Product.vue file:
Aside from the product container section, we introduced and used a <comet-chat-messages></comet-chat-messages> components to render messages as the list of conversations between the logged in user who can also be called the buyer and the product owner, in this case, the seller. This component was imported from the CometChat UI kit for Vuejs and it takes the type, logged-in-user and item as props. The props are very important as this component will throw an error without them.
Once this component is mounted, we called the CometChat.getUser() to retrieve the details of the authenticated user and the product owner object and assigned them to both the logged-in-user and item props respectively.
To make this page layout more appealing and properly structure, use the following style for the <style></style> section of the file:
Add Inbox Page
Open the Inbox.vue file and use the following code to show the list of conversations between buyers and a seller:
Here, we once again leverage the CometChat Vue UI kit and imported CometChatConversationListWithMessages component from it. This component already contains all the necessary listeners and methods required to display the recent conversation list and shows the set of the messages or chats of the selected recent conversations.
Create a product

**Conclusion
**
Here, we built a marketplace site with Laravel, Vuejs and CometChat. Implementing a chat functionality in a new or existing application is always a tedious task to complete. Leveraging CometChat infrastructure here in this tutorial makes it so easy. With an existing UI components that contains the required logic and listeners for a functional chat app, you don’t have to hit your head on the wall trying to figure out what to do. Get in touch with our support experts if you have any questions or need help with this tutorial.
The complete source code for the project built in this tutorial can be found here on GitHub.
Join our newsletter for more tutorials and content like this delivered straight to your inbox. Here are a some suggestions we think you will love -
tutorial 1
tutorial 2
tutorial 3
About the author
Oluyemi is a tech enthusiast with a background in Telecommunication Engineering. He ventured into programming with a keen interest in solving day-to-day problems encountered by users. He has since directed his problem-solving skills towards building software for both the web and mobile platforms. As a full-stack software engineer with a passion for sharing knowledge, Oluyemi has published many technical articles and content on several blogs worldwide. As s tech-savvy person, his hobbies include trying out new programming languages and frameworks.
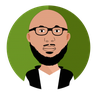
Olususi Oluyemi
CometChat