This tutorial is outdated. We're currently working on an updated version. In the meantime, for instructions on building a chat app using CometChat, please visit our documentation.
App and web development have come a long way over the last few years. We use a lot of social apps every day, including Messenger, WhatsApp, Snapchat and so on. One of the most widely used features is live chat and voice/video chat. Using the Android Kotlin, CometChat Android UI Kit, Firebase backend services, you will learn how to build an Instagram clone with minimal effort.
We should cover the following topics in this tutorial:
1. Authentication
A way for users to log in and register
Login the logged-in user to CometChat
Create a new user by using the Firebase Authentication service, and then use the CometChat SDK to create a new CometChat user.
User superhero dummy users for this tutorial
2. User Profiles
A tab to display user details.
Display all posts made by the user.
3. Follow Requests
Users can follow other users by simply clicking a ‘Follow’ button on any users profile
4. Create New Post
Functionality to upload new image/video as post
5. Feed
A tab where users can see content uploaded by other users they follow
Functionality to Like posts
6. Reels
Ability for users to upload short (1-min) videos
Display these reels in the Feeds tab
7. Notifications
Notifications for new messages, likes and follow requests
A tab where users can see all the latest notifications.
8. Chat
Ability to text all other users
Voice & Video calling
Reactions, Media sharing, and read receipts
This tutorial will use the Android Kotlin, CometChat Android UI Kit, Firebase backend services to build the Instagram clone.
Prerequisites
To follow this tutorial, you must have a degree of understanding of the general use of Android. In this project, we will use Android Studio to build the Android app. This will help you to improve your understanding of this tutorial.
Integrate CometChat Android UI Kit
To integrate CometChat Android UI Kit, you need to follow the below steps:

Project Structure after adding the CometChat UI Kit
Step 1: Clone the UI kit from android-chat-ui-kit-repository.
Step 2: Import uikit Module from Module Settings.( To know how to import uikit as Module visit this link),
Step 3: Re-check your folder structure. If the library is added successfully it will look like the above image
Step 4: Add the following parts to your build.gradle file (app level).
android { … dataBinding { enabled = true } … defaultConfig { ... manifestPlaceholders = [file_provider: "YOUR_PACKAGE_NAME"] //add your application package. } }
After integrating CometChat Android UI Kit successfully, you need to use the following statement to open the CometChat UI Screen. CometChatUI is a way to launch a fully working chat application using the UI Kit .In UI Unified all the UI Screens and UI Components working together to give the full experience of a chat application with minimal coding effort.
Installing the App Dependencies
Step 1: you need to have Java - JDK 8 and Android Studio installed on your machine
Step 2: create a new project with the name Instagram Clone by using Android Studio.
Step 3: Replace your dependencies inside build.gradle file (app level) with the following part.
implementation 'androidx.core:core-ktx:1.3.2' implementation 'androidx.appcompat:appcompat:1.3.1' implementation 'com.google.android.material:material:1.4.0' implementation 'androidx.constraintlayout:constraintlayout:2.1.1' implementation platform('com.google.firebase:firebase-bom:29.0.0') implementation 'com.google.firebase:firebase-analytics-ktx' implementation 'com.google.firebase:firebase-auth-ktx' implementation 'com.google.firebase:firebase-database-ktx' implementation 'com.google.firebase:firebase-storage-ktx' implementation 'com.cometchat:pro-android-chat-sdk:3.0.0' implementation 'com.cometchat:pro-android-calls-sdk:2.1.0' implementation "androidx.recyclerview:recyclerview:1.2.1" implementation 'com.github.bumptech.glide:glide:4.12.0' implementation project(path: ':uikit-kotlin') implementation 'androidx.legacy:legacy-support-v4:1.0.0' annotationProcessor 'com.github.bumptech.glide:compiler:4.12.0'
*Note: In this project, we use the RecyclerView to show the list of data, and use the Glide library to show the image. The Glide library helps us to achieve some effects that we need in this application such as circle-crop, center-crop the images.
Step 4: Add the repository URL to the project level build.gradle file in the repositories block under the all projects section
allprojects { repositories { maven { url "https://dl.cloudsmith.io/public/cometchat/cometchat-pro-android/maven/" } } }
*Note: If you are using Android Studio Arctic Fox 2020.3.1, the repository URL should be included in the settings.gradle file not the build.gradle file (project level).
Step 5: Inside your build.gradle file (app level), please add the following section.
android { compileOptions { sourceCompatibility JavaVersion.VERSION_1_8 targetCompatibility JavaVersion.VERSION_1_8 } }
Step 6: Sync the project.
Configuring CometChat SDK
Head to CometChat Dashboard and create an account.

Register a new CometChat account if you do not have one
Add real-time chat with minimal effort using CometChat
2.After registering a new account, you need to the log in to the CometChat dashboard.

Log in to the CometChat Dashboard with your created account
3. From the dashboard, add a new app called "instagram-clone".

Create a new CometChat app - Step 1

Create a new CometChat app - Step 2
4. Select this newly added app from the list.

Select you created app
5. From the Quick Start copy the APP_ID, REGION, and AUTH_KEY, which will be used later.

Copy the the APP_ID, REGION, and AUTH_KEY
6. Navigate to the Users tab, and delete all the default users (very important).

Navigate to Users tab and delete all the default users
7. Navigate to the Groups tab and delete all the default groups (very important).

Navigate to Group tab and delete all the default groups
8.Create a file called Constants.kt in the package folder of your project.
9. Import and inject your secret keys in the Constants.kt file containing your CometChat and Firebase in this manner.
10. Make sure to include the Constants.kt file in your gitIgnore file from being exposed online.
Setting Up Firebase Project
According to the requirements of the Instagram clone, you need to let users create a new account and login to the application, Firebase will be used to achieve that. Head to Firebase to create a new project and activate the email and password authentication service. This is how you do it:
To begin using Firebase, you’ll need a Gmail account. Head over to Firebase and create a new project.

Firebase
Firebase provides support for authentication using different providers. For example, Social Auth, phone numbers, as well as the standard email and password method. Since you will be using the email and password authentication method in this tutorial, you need to enable this method for the project you created in Firebase, as it is by default disabled.
Under the authentication tab for your project, click the sign-in method and you should see a list of providers currently supported by Firebase.
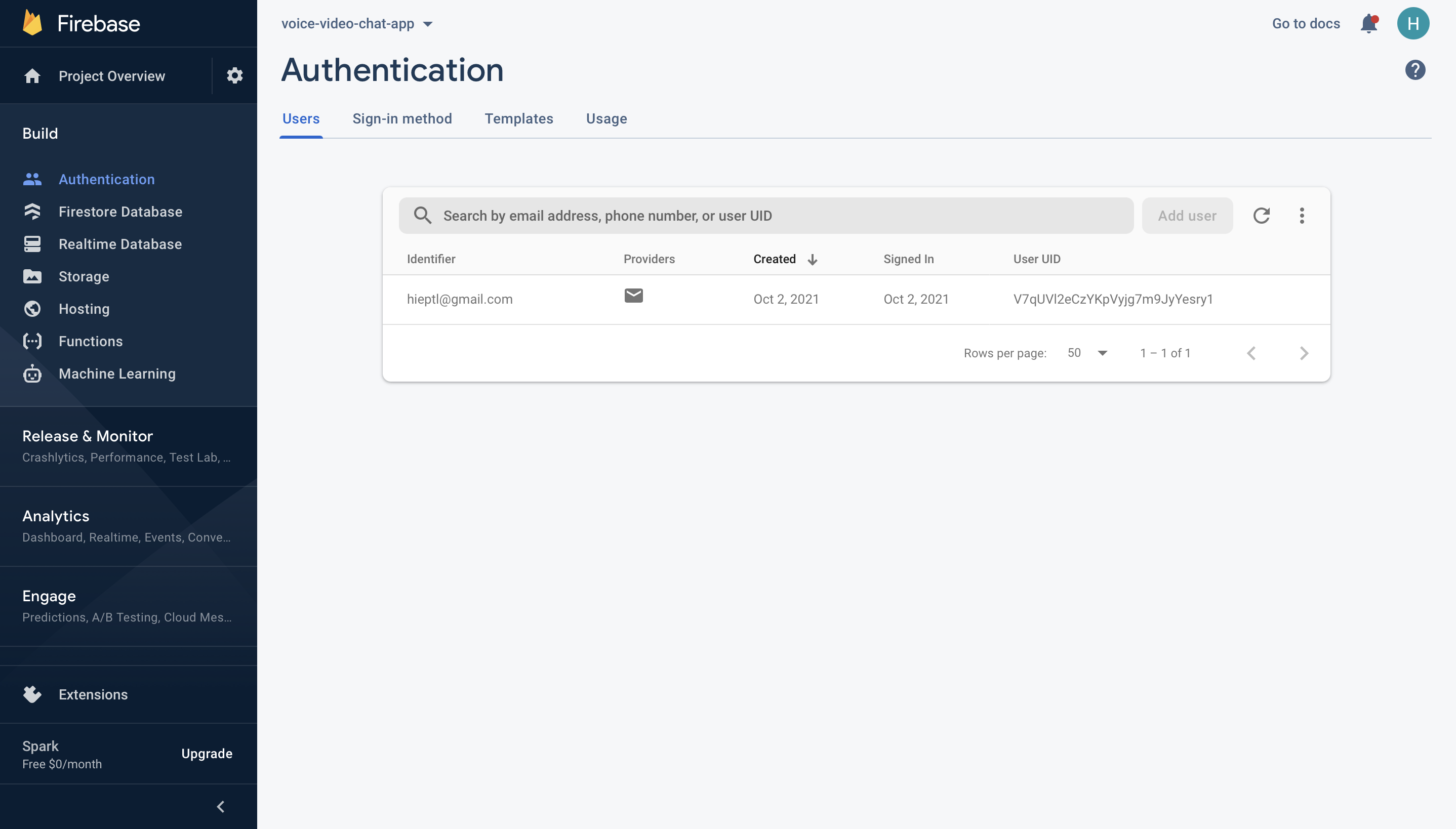
Firebase Authentication
Next, click the edit icon on the email/password provider and enable it.

Firebase Authentication with Email and Password
Now, you need to go enable Firebase Realtime Database. We will be using Firebase Realtime Database to store the information of the users in the application. Please refer to the following part for more information.
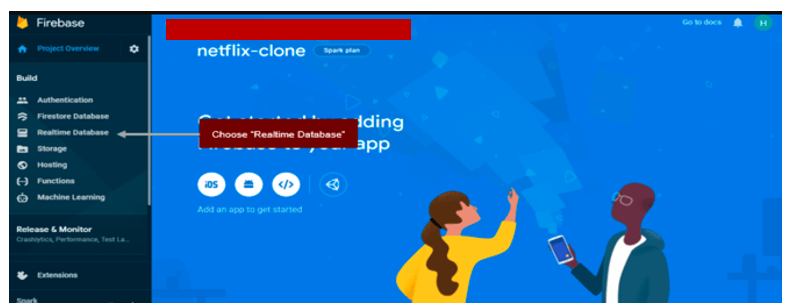
Choose “Realtime Database” option

Click on “Create Database"

Select location where you realtime database will be stored
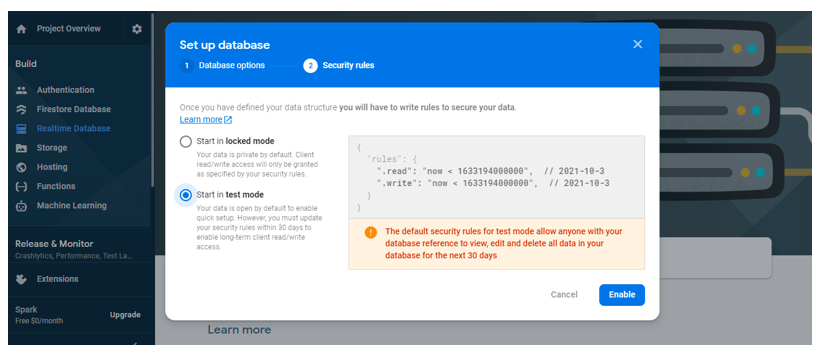
Select “Start in test mode” for the learning purpose
Please follow the guidance from Firebase. After following all steps, you will see the database URL. If you just need to update the “FIREBASE_REALTIME_DATABASE_URL” variable in your “Constants.kt” file with that value.
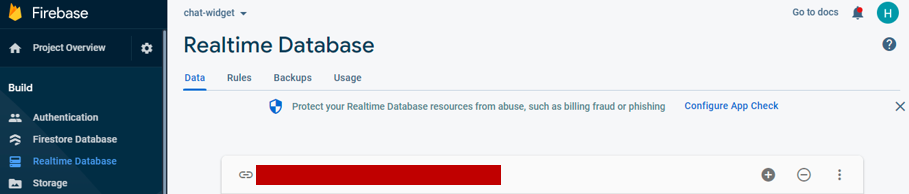
Database URL
On the other hand, your Firebase real-time database will be expired in the future. To update the rules you just need to select the “Rules” tab and update the date/time in milliseconds as you can see in the image below.

Update Firebase Realtime Database Rules
In this project, we also need to upload the post’s images, and user’s avatars. Therefore, we have to enable the Firebase Storage service, the Firebase Storage service helps us store the assets files including images, videos and so on. To enable the Firebase Storage service, please follow the below steps

Enable Firebase Storage Service

Click on the “Next” button

Click on the “Done” button

Update the rules
For the learning purposes, we need to update the rules of the Firebase Storage service. It means that everyone, who can access to the application, can upload to the Firebase storage.
To set up Firebase for the Android app, we can use Firebase Assistance. You need to follow the below steps to enable Firebase Assistance inside your Android Studio.
Step 1: Click Tools and then choose “Firebase”.

Enable Firebase Assistance - Step 1
Step 2: Open the assistance by click on the “Assistance” on the right of your Android Studio.

Enable Firebase Assistance - Step 2
Step 3: You can expand “Authentication” part, “Realtime Database” part, “Cloud Storage for Firebase” part, and follow the tutorials from Firebase Assistance.
The below images demonstrate the data structures for users, posts, and notifications. The user information contains the avatar, email, name, number of followers, the list of followers, number of posts, and uid.

Data Structure - User
On the other hand, the post contains the information of the author, content, id, list of likes, number of likes and post’s category.

Data Structure - Post
The notification information contains the notification’s id, notification’s image, notification’s message, and receiver’s id.

Data Structure - Notification
Configuring Strings and Styling, Themes for the Application
Inside our Instagram clone project structure, you need to follow the below steps to create strings, styling and themes with the below steps, by doing this, we can save time and speed up the development process. We will focus on the business logic in the following section.
Copy the values from here and then paste to your “strings.xml” file.
Copy the values from here and then paste to your “colors.xml” file.
Copy the values from here and then paste to your “themes.xml” file.
Configuring Drawables for the Application
We will focus on the business logic in this application. For this reason, to get the the drawables, you can refer to this link. The drawable folder will contain images, shape and so on for the application.
Configuring Layouts for the Application
In the project structure, the layout folder contains all layouts that we need for the Instagram clone including the layouts for activities, fragments, recycler view item, and so on. Please refer to this link to get the layout for the application.
The Login Page

The Login Activity
This component is responsible for authenticating our users using the Firebase google authentication service. It accepts the user credentials and either signs him up or in, depending on if he is new to our application.
The image describes that the user needs to input the email and password. To log in to the application, the user needs to click on the “Login” button. The application will validate the user’s credentials first and then call Firebase Authentication Service. If everything is fine, the application will also let the user log in to the CometChat as well. The code logic for the Login Activity can be found here. You can refer to the below code snippet for more information.
As mentioned above, if the user’s credentials are valid, we will call the Firebase Authentication service and the CometChat service. Moreover, we need to initialize CometChat in our application and we also call the “loginCometChat” function, this function will take responsibility for letting the user access the CometChat. You can refer to the below code snippet for more information.
According the the UI, the user can go to the sign up page to create a new account by clicking on the “Register” text. The sign up page will be discussed in the following section.
The Sign Up Page

The SignUp Activity
According to the requirements, the application needs to provide a way to let the users create new accounts. For this reason, the sign-up page needs to be created to achieve that. From the login page, after the user clicks on the “Register” text, the user will be redirected to the sign-up page. As you can see on the beside image, the user needs to input some information to create a new account such as the user’s avatar, user’s name, user’s email, user’s password, and confirm password.
The last but not least, to create a new account, the final step is to click on the “Register” button. After clicking the button, the application will register a new account by using the Firebase Authentication service, upload the user’s avatar to the Firebase Cloud Storage, and insert new user’s information to the Firebase Realtime Database and then register a new account on CometChat.
The code logic for the SignUpActivity can be found here. You can refer to the below code snippet for more information.
What we have done in the “SignUpActivity.kt” will be similar to the “LoginActivity.kt”. After the user inputs enough information and then clicks on the “Register” button, the “registerCometChatAccount” will be dispatched. You can refer to the below code snippet for more information.
The Main Activity

The Main Activity
After the users have logged in to the application, the user will be redirected to the main page. In this page, we have the bottom navigation view with three fragments - The feed fragment, the notification fragment, and the profile fragment. You can create three empty fragments with the corresponding name - FeedFragment, Notification Fragment, ProfileFragment to make your code buildable. Aside from that, the Main Activity also contains the header of the application including the logo, the plus icon, the power icon, and the chat icon. If the users click on the power icon, a confirmation dialog will be shown to make sure that the users would like to log out from the application. Following that, if the users click on the chat icon, the users will be redirect to the chat page. Last but not least, the user can click on the plus icon to go to the CreateActivity so the users can create and upload new post. The source code can be found here.
The Create Activity

The Create Activity
As mentioned above, the users click on the plus icon to go the Create Activity. In the Create Activity, the users can create and upload new post. The source code can be found here.
The Feed Fragment

The Feed Fragment
The Feed Fragment is used to show the first tab of the bottom navigation view. It interacts with the Firebase Realtime Database service to get the list of posts. The full source code of the Feed Fragment can be found here.
In the Feed Fragment, to show the list posts, we use the RecyclerView. Therefore, we need to define a adapter for the RecyclerView. Please create a file called “PostAdapter.kt”. The full source code of the “PostAdapter.kt” file can be found here.
The Detail Activity
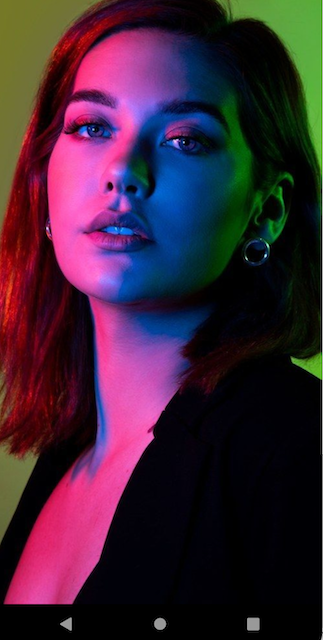
The Detail Activity
After the users click on the image of any post on the list, the users will be redirected to the Detail Activity. The Detail Activity is used to show the detail information of the selected post. Please create the “DetailActivity.kt” file. The full source code of the “DetailActivity.kt” file can be found here.
The Notifications Fragment

The Notifications Fragment
After the users like any post or follow someone, the notification will be created for the related users, and save to the Firebase Realtime Database. The Notification Fragment interacts with the Firebase Realtime Database to get the list of notifications that are belonging to the current user. The full source code of the Notification Fragment can be found here.
To show the list of notifications, we use the RecyclerView. Hence, we create to create a adapter for it. Please create a file call “NotificationAdapter.kt”.
The Profile Fragment

The Profile Fragment
The Profile Fragment is used to show the current user information. The Profile Fragment gets the CometChat aid and then call the Firebase Realtime Database to get the user detail information. On the other hand, the Profile Fragment also call the Firebase Realtime Database to get the list of posts that are belonging to that user. The full source code can be found here.
To show the list of posts of the current user, we use the RecyclerView. We need to create a adapter for the RecyclerView, please create a file called “ProfilePostAdapter.kt”. The full source code can be found here.
The CometChat UI Activity

The CometChatUI Activity
According to the CometChat documentation, to launch to CometChat UI, we need to run the following statement:
In the Instagram clone, the users can go to the chat page by clicking on the chat icon of the header. You can refer to the below code snippet for more information.
Customize the CometChat UI

Customize the CometChat UI
The default UI of the CometChat UI kit contains the bottom navigation, and does not contain the header. To achieve the UI as you can see in the above image, we need to customize the UI Kit. We need to modify the CometChatUI.kt as follow:
Aside from that, we need to update the activity_cometchat_unified.xml and cometchat_navigation_bottom.xml files.
After the users click on the “more” icon we need to show the user profile activity. For this reason, we need to create the “UserProfileActivity.kt” file inside the “userProfile” folder. The full source code of the “UserProfileActivity.kt” file can be found here, and its XML can be found here.
Wrapping Up
In conclusion, we have done an amazing job in developing an Instagram clone by leveraging Android, Firebase, and CometChat. You’ve been introduced to the chemistry behind the Instagram clone and how the CometChat Android UI Kit makes chat applications buildable.
You have seen how to integrate most of the CometChat functionalities such as texting and real-time messaging. I hope you enjoyed this tutorial and that you were able to successfully build the Instagram clone. It's time to get busy and build other related applications with the skills you have gotten from this tutorial. You can start building your chat app for free by signing up to the cometchat dashboard here.
About the Author
Hiep Le is a software engineer. He takes a huge interest in building software products and is a full-time software engineer. Most of his work is focused on one thing - to help people learn.
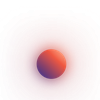
Hiep Le
CometChat